如何使用python调用mysql
时间: 2024-01-27 14:06:06 浏览: 57
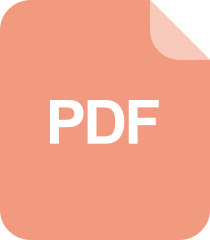
mysql for python
使用Python调用MySQL需要安装Python的MySQL驱动程序,这里以`mysql-connector-python`为例:
1. 首先安装`mysql-connector-python`模块,可以在命令行中输入以下命令:
```
pip install mysql-connector-python
```
2. 然后在Python代码中导入`mysql.connector`模块:
```python
import mysql.connector
```
3. 创建MySQL连接对象,并使用连接对象创建游标对象:
```python
# 创建连接
conn = mysql.connector.connect(user='your_username', password='your_password', host='your_host', database='your_database')
# 创建游标
cursor = conn.cursor()
```
4. 执行SQL语句并获取结果:
```python
# 执行SQL语句
cursor.execute('SELECT * FROM your_table')
# 获取结果
result = cursor.fetchall()
```
5. 关闭游标和连接:
```python
# 关闭游标
cursor.close()
# 关闭连接
conn.close()
```
完整的代码示例:
```python
import mysql.connector
# 创建连接
conn = mysql.connector.connect(user='your_username', password='your_password', host='your_host', database='your_database')
# 创建游标
cursor = conn.cursor()
# 执行SQL语句
cursor.execute('SELECT * FROM your_table')
# 获取结果
result = cursor.fetchall()
# 输出结果
for row in result:
print(row)
# 关闭游标
cursor.close()
# 关闭连接
conn.close()
```
注意:在实际使用中,需要替换`your_username`、`your_password`、`your_host`、`your_database`、`your_table`等参数为实际的值。
阅读全文
相关推荐
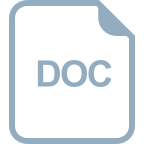
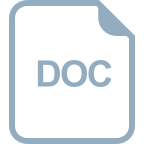
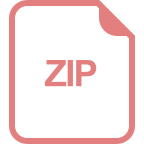



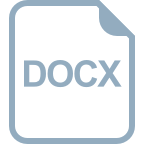
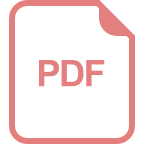
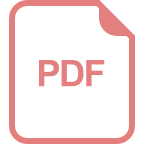
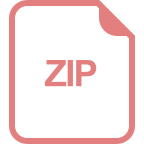






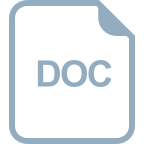
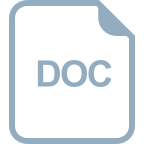