helloworldgame字符串随鼠标移动
时间: 2024-10-09 13:01:22 浏览: 21
"HelloWorldGame" 字符串随着鼠标移动通常是指一种简单的交互式界面设计,在这种游戏中,用户可以通过鼠标操作控制屏幕上的 "Hello World" 文本跟随鼠标的移动。这可能是通过前端网页技术(如HTML5、JavaScript),或者游戏开发引擎(如Unity、Unreal Engine)结合CSS动画来实现的。开发者会监听鼠标的坐标变化,并实时更新文本的位置,使其看起来像是随着鼠标移动。
例如,在HTML5中,可以这样实现:
```html
<!DOCTYPE html>
<html>
<body>
<div id="text">Hello World</div>
<script>
document.getElementById("text").style.position = 'absolute';
window.addEventListener('mousemove', function(e) {
document.getElementById("text").style.left = e.clientX + 'px';
document.getElementById("text").style.top = e.clientY + 'px';
});
</script>
</body>
</html>
```
在这个例子中,当鼠标移动时,`Hello World` 文本的位置会根据 `e.clientX` 和 `e.clientY` 更新,模拟跟随鼠标。
相关问题
c语言字符串移动完整代码
在C语言中,如果你想移动一个字符串,通常不需要直接操作内存地址,因为字符串在C语言中是字符数组。如果你想要将一个字符串复制到另一个位置,可以使用标准库函数`strcpy()`或者手动遍历字符串并逐个赋值。以下是两个例子:
**使用`strcpy()`函数的例子:**
```c
#include <stdio.h>
#include <string.h>
void move_string(char *dest, const char *src) {
// 检查目标字符串是否足够大容纳源字符串
if (strlen(src) + 1 > strlen(dest)) {
printf("Destination string is too small.\n");
return;
}
// 移动字符串内容
strcpy(dest, src);
}
int main() {
char source[] = "Hello, World!";
char destination[20]; // 假设destination有足够空间
move_string(destination, source);
printf("Source: %s\n", source);
printf("Destination after move: %s\n", destination);
return 0;
}
```
**手动复制的例子:**
```c
#include <stdio.h>
void manual_move(char *dest, const char *src) {
int i;
for (i = 0; src[i] != '\0'; i++) {
dest[i] = src[i];
}
dest[i] = '\0'; // 添加终止符
}
// 使用同上...
```
在以上两个示例中,`move_string()`和`manual_move()`函数都是将`src`字符串的内容复制到了`dest`。注意在使用`strcpy()`时要检查目标字符串的空间。
向右移动字符串c语言
以下是一个向右移动字符串的C语言例子:
```c
#include <stdio.h>
#include <string.h>
void right_move(char* str, int k) {
int len = strlen(str);
k = k % len;
char tmp[k];
strncpy(tmp, str + len - k, k);
memmove(str + k, str, len - k);
strncpy(str, tmp, k);
}
int main() {
char str[] = "abcdefg";
int k = 3;
right_move(str, k);
printf("%s\n", str); // 输出:efgabcd
return 0;
}
```
该例子中,我们定义了一个`right_move`函数,该函数接受一个字符串和一个整数k作为参数,表示将字符串向右移动k个字符。我们首先计算出字符串的长度,并将k对字符串长度取模,以避免k大于字符串长度的情况。然后,我们定义一个长度为k的字符数组tmp,将原字符串中最后k个字符复制到tmp中。接着,我们使用`memmove`函数将原字符串中前面的字符向右移动k个位置,最后将tmp中的字符复制到原字符串的前k个位置。最后,我们在main函数中调用`right_move`函数,并输出移动后的字符串。
阅读全文
相关推荐
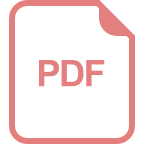
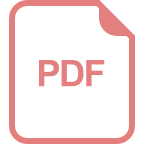
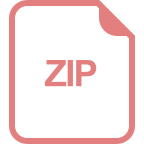













