ReentrantLock 代码示例
时间: 2023-10-03 20:01:47 浏览: 72
Sure, here is an example of using ReentrantLock in Java:
```
import java.util.concurrent.locks.ReentrantLock;
public class LockExample {
private final ReentrantLock lock = new ReentrantLock();
public void doSomeWork() {
lock.lock();
try {
// critical section
// this code will only be executed by one thread at a time
} finally {
lock.unlock();
}
}
}
```
In this example, the `lock` object ensures that only one thread can execute the critical section of code at a time. The `lock()` method is called to acquire the lock, and the `unlock()` method is called to release it.
相关问题
ReentrantLock 锁代码示例
以下是 ReentrantLock 锁的代码示例:
```java
import java.util.concurrent.locks.ReentrantLock;
public class Counter {
private int count = 0;
private ReentrantLock lock = new ReentrantLock();
public void increment() {
lock.lock(); // 获得锁
try {
count++; // 执行操作
} finally {
lock.unlock(); // 释放锁
}
}
public int getCount() {
return count;
}
}
```
在上面的示例中,我们使用 ReentrantLock 类来保护 Counter 类中的 count 变量。在 increment() 方法中,我们首先调用 lock() 方法来获得锁,然后执行 count++ 操作,最后调用 unlock() 方法来释放锁。这样,我们就可以保证 count 变量的线程安全性。
如何使用ReentrantLock和Condition接口实现Java中的生产者消费者模式,并提供相应的代码示例?
在Java中实现生产者消费者模式时,除了传统的`synchronized`和`wait/notify`机制外,还可以利用`ReentrantLock`和`Condition`接口来更精确地控制线程间的协作。这种方式提供了更大的灵活性和更强的功能,允许线程在不同的条件变量上等待,并且可以设置不同的唤醒策略。以下是使用`ReentrantLock`和`Condition`实现生产者消费者模式的示例代码:
参考资源链接:[Java并发编程:线程间通信与协作](https://wenku.csdn.net/doc/1gc523mjtp?spm=1055.2569.3001.10343)
```java
import java.util.LinkedList;
import java.util.Queue;
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.ReentrantLock;
public class ProducerConsumerExample {
private Queue<Integer> queue = new LinkedList<>();
private int maxSize = 10;
private ReentrantLock lock = new ReentrantLock();
private Condition notFull = lock.newCondition();
private Condition notEmpty = lock.newCondition();
public void produce(int item) throws InterruptedException {
lock.lock();
try {
while (queue.size() == maxSize) {
notFull.await();
}
queue.offer(item);
System.out.printf(
参考资源链接:[Java并发编程:线程间通信与协作](https://wenku.csdn.net/doc/1gc523mjtp?spm=1055.2569.3001.10343)
阅读全文
相关推荐
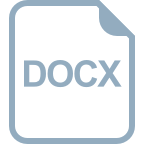
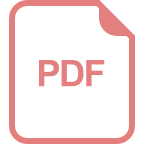
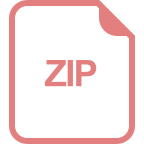
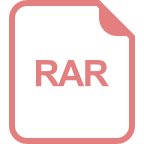
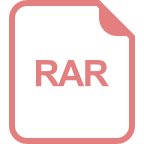
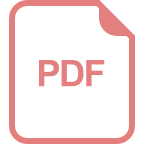
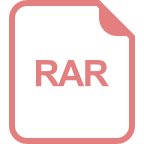
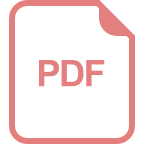
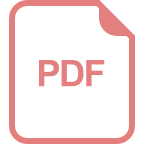
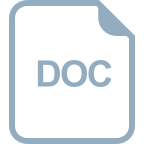
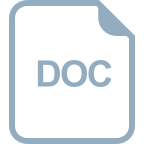
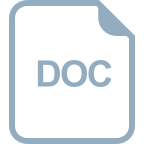
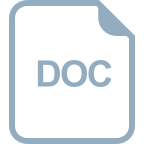
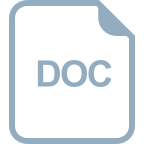
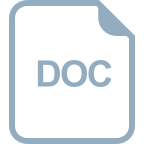
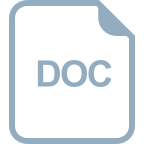
