java多线程上传文件
时间: 2023-10-29 12:06:34 浏览: 43
以下是使用Java多线程上传文件的示例代码:
```java
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class FileUploader implements Runnable {
private File file;
private String url;
public FileUploader(File file, String url) {
this.file = file;
this.url = url;
}
@Override
public void run() {
try {
URL serverUrl = new URL(url);
HttpURLConnection connection = (HttpURLConnection) serverUrl.openConnection();
connection.setRequestMethod("POST");
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type", "application/octet-stream");
connection.setRequestProperty("Content-Disposition", "attachment; filename=\"" + file.getName() + "\"");
connection.setRequestProperty("Content-Length", String.valueOf(file.length()));
byte[] buffer = new byte[4096];
int bytesRead = -1;
int bytesSent = 0;
BufferedInputStream inputStream = new BufferedInputStream(new FileInputStream(file));
OutputStream outputStream = connection.getOutputStream();
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
bytesSent += bytesRead;
}
outputStream.flush();
outputStream.close();
inputStream.close();
if (connection.getResponseCode() == HttpURLConnection.HTTP_OK) {
System.out.println("File uploaded successfully.");
} else {
System.out.println("Server returned HTTP response code: " + connection.getResponseCode());
}
connection.disconnect();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
```
你可以在主类中创建多个线程,每个线程上传一个文件:
```java
public class Main {
public static void main(String[] args) {
String url = "http://example.com/upload";
File file1 = new File("file1.txt");
File file2 = new File("file2.txt");
Thread thread1 = new Thread(new FileUploader(file1, url));
Thread thread2 = new Thread(new FileUploader(file2, url));
thread1.start();
thread2.start();
}
}
```
注意,这只是一个简单的示例,你还需要添加异常处理和其他必要的安全检查。
相关推荐
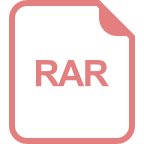














