使用qcustomplot画两个折线图
时间: 2023-12-25 21:06:09 浏览: 28
使用qcustomplot画两个折线图的步骤如下:
1. 在Qt项目中添加qcustomplot库。
2. 创建一个QWidget对象,作为绘图区域。
3. 创建两个QVector对象,用于存储折线图的数据。
4. 创建一个QCustomPlot对象,并设置绘图区域的范围和标题。
5. 创建两个QCPGraph对象,分别绑定到QVector对象上,并设置折线图的颜色、宽度等属性。
6. 将两个QCPGraph对象添加到QCustomPlot对象中。
7. 调用QCustomPlot对象的replot()函数,进行绘图。
下面是一个简单的示例代码:
```
#include <QApplication>
#include <QWidget>
#include "qcustomplot.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
// 创建QWidget对象作为绘图区域
QWidget *widget = new QWidget();
widget->setFixedSize(400, 300);
// 创建两个QVector对象存储折线图数据
QVector<double> x(101), y1(101), y2(101);
for (int i=0; i<101; i++)
{
x[i] = i/10.0;
y1[i] = qSin(x[i]);
y2[i] = qCos(x[i]);
}
// 创建QCustomPlot对象,并设置范围和标题
QCustomPlot *plot = new QCustomPlot(widget);
plot->setGeometry(10, 10, 380, 280);
plot->plotLayout()->insertRow(0);
plot->plotLayout()->addElement(0, 0, new QCPTextElement(plot, "My Plot", QFont("sans", 12, QFont::Bold)));
plot->xAxis->setLabel("x");
plot->yAxis->setLabel("y");
plot->xAxis->setRange(0, 10);
plot->yAxis->setRange(-1, 1);
// 创建两个QCPGraph对象,并绑定到QVector对象上
QCPGraph *graph1 = plot->addGraph();
graph1->setData(x, y1);
graph1->setPen(QPen(Qt::red));
graph1->setLineStyle(QCPGraph::lsLine);
graph1->setScatterStyle(QCPScatterStyle(QCPScatterStyle::ssCircle, 5));
QCPGraph *graph2 = plot->addGraph();
graph2->setData(x, y2);
graph2->setPen(QPen(Qt::blue));
graph2->setLineStyle(QCPGraph::lsLine);
graph2->setScatterStyle(QCPScatterStyle(QCPScatterStyle::ssSquare, 5));
// 进行绘图
plot->replot();
widget->show();
return a.exec();
}
```
运行程序,会显示一个大小为400x300的窗口,其中包含两个折线图。其中,红色折线图为y=sin(x),蓝色折线图为y=cos(x)。
相关推荐
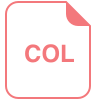
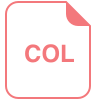
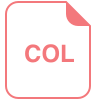
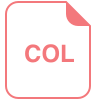
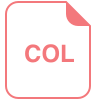









