stm32f103c8t6使用nrf24l01控制led的开关代码
时间: 2023-02-11 07:12:37 浏览: 153
STM32F103C8T6使用NRF24L01控制LED的代码如下:
```
#include "stm32f10x.h"
#include "nrf24l01.h"
#include "led.h"
int main(void)
{
NRF_Init();
LED_Init();
while(1)
{
if(NRF_Read_IRQ())
{
if(NRF_RX_DR())
{
if(NRF_Read_Buf(NRF_Read_Payload()) == 0x01)
{
LED_On();
}
else
{
LED_Off();
}
}
}
}
}
```
请注意,在这里假设你已经有nrf24l01和led驱动程序的代码。
需要根据你自己的实际情况来调整驱动程序。
相关问题
stm32f103c8t6用nrf24l01代码
以下是一个基于stm32f103c8t6和nrf24l01的简单代码示例,可以用于发送和接收数据:
```c
#include "stm32f10x.h"
#include "nrf24l01.h"
#define NRF_CE_HIGH() GPIO_SetBits(GPIOA, GPIO_Pin_4)
#define NRF_CE_LOW() GPIO_ResetBits(GPIOA, GPIO_Pin_4)
int main(void)
{
// 初始化nrf24l01
nRF24L01_Init();
// 设置nrf24l01为接收模式
nRF24L01_RX_Mode();
while(1)
{
// 检查是否有数据接收到
if(nRF24L01_RxPacket(RxMessage) == RX_OK)
{
// 处理接收到的数据
// ...
}
}
}
// 发送数据
void send_data(uint8_t *buf, uint8_t len)
{
// 设置nrf24l01为发送模式
nRF24L01_TX_Mode();
// 发送数据
nRF24L01_TxPacket(buf, len);
// 等待发送完成
while(nRF24L01_Get_Status() & (1 << TX_DS));
// 设置nrf24l01为接收模式
nRF24L01_RX_Mode();
}
```
需要注意的是,此示例代码中使用了nrf24l01库文件,你需要将其加入到你的工程中。另外,此代码中使用了PA4作为nrf24l01的CE引脚,你可以根据你的实际情况进行修改。
基于STM32F103C8T6的NRF24L01驱动代码
您好!对于基于STM32F103C8T6的NRF24L01驱动代码,以下是一个简单的示例:
```c
#include "stm32f10x.h"
#include "nrf24l01.h"
void NRF24L01_Init(void)
{
// 初始化SPI接口
SPI_InitTypeDef SPI_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
// 打开SPI和GPIO的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SPI1 | RCC_APB2Periph_GPIOA, ENABLE);
// 配置SPI引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_10MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置SPI参数
SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_Low;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_1Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_8;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_Init(SPI1, &SPI_InitStructure);
// 使能SPI
SPI_Cmd(SPI1, ENABLE);
// 初始化NRF24L01
NRF24L01_InitSPI(); // 初始化SPI接口
NRF24L01_CE_LOW(); // 先将CE拉低
NRF24L01_CSN_HIGH(); // 先将CSN拉高
}
void NRF24L01_SendData(uint8_t* tx_buf, uint8_t len)
{
NRF24L01_CE_LOW(); // 先将CE拉低
// 设置为发送模式
NRF24L01_WriteReg(NRF24L01_CONFIG, NRF24L01_CONFIG_MODE_TX);
// 清除发送完成中断标志
NRF24L01_ClearIRQFlags(NRF24L01_IRQ_FLAG_TX_DS);
// 写入发送数据
NRF24L01_WritePayload(tx_buf, len);
// 启动发送
NRF24L01_CE_HIGH();
}
void NRF24L01_ReceiveData(uint8_t* rx_buf, uint8_t* len)
{
if (NRF24L01_GetStatus() & NRF24L01_STATUS_RX_DR)
{
NRF24L01_CE_LOW(); // 先将CE拉低
// 读取接收到的数据
NRF24L01_ReadPayload(rx_buf, len);
// 清除接收完成中断标志
NRF24L01_ClearIRQFlags(NRF24L01_IRQ_FLAG_RX_DR);
// 设置为接收模式
NRF24L01_WriteReg(NRF24L01_CONFIG, NRF24L01_CONFIG_MODE_RX);
// 启动接收
NRF24L01_CE_HIGH();
}
}
int main(void)
{
uint8_t tx_buf[] = "Hello, NRF24L01!";
uint8_t rx_buf[32];
uint8_t rx_len;
// 初始化NRF24L01
NRF24L01_Init();
while (1)
{
// 发送数据
NRF24L01_SendData(tx_buf, sizeof(tx_buf));
// 接收数据
NRF24L01_ReceiveData(rx_buf, &rx_len);
// 处理接收到的数据
if (rx_len > 0)
{
// TODO: 处理接收到的数据
rx_buf[rx_len] = '\0'; // 添加字符串结束符号
printf("Received: %s\n", rx_buf);
}
}
}
```
请注意,上述代码仅为示例代码,您可能需要根据您的具体需求进行适当的修改和调整。此外,您还需要在项目中添加相应的头文件和函数定义,以及适配您的硬件配置和引脚连接。
希望对您有所帮助!如有任何问题,请随时提问。
相关推荐
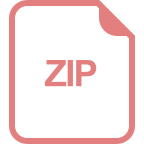
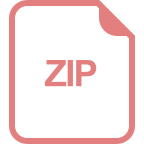
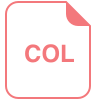
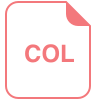
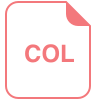
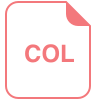
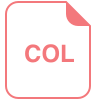







