openmv 识别颜色(黄色,蓝色,绿色),形状(长方形,圆形,五角星,三角形)代码
时间: 2023-08-19 10:03:51 浏览: 64
下面是一个简单的OpenMV代码,用于识别颜色和形状:
```
import sensor, image, time
from pyb import UART
# 颜色阈值
yellow_threshold = (43, 100, -33, 18, 8, 62)
blue_threshold = (0, 100, -128, 80, -128, -10)
green_threshold = (10, 60, -50, -20, -30, 60)
# 形状阈值
rect_threshold = 0.2
circle_threshold = 0.8
star_threshold = 0.2
triangle_threshold = 0.2
# 初始化串口
uart = UART(3, baudrate=9600, timeout_char=1000)
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
sensor.set_auto_gain(False)
sensor.set_auto_whitebal(False)
# 主循环
while(True):
img = sensor.snapshot()
# 识别黄色
blobs = img.find_blobs([yellow_threshold])
for blob in blobs:
# 形状检测:长方形
if blob.w() / blob.h() > 1 - rect_threshold and blob.w() / blob.h() < 1 + rect_threshold:
uart.write("Yellow rectangle\n")
# 形状检测:圆形
elif blob.circularity() > circle_threshold:
uart.write("Yellow circle\n")
# 形状检测:五角星
elif blob.corners() == 5 and blob.area() > 500 and blob.area() < 5000:
uart.write("Yellow star\n")
# 形状检测:三角形
elif blob.corners() == 3 and blob.area() > 500 and blob.area() < 5000:
uart.write("Yellow triangle\n")
# 识别蓝色
blobs = img.find_blobs([blue_threshold])
for blob in blobs:
# 形状检测:长方形
if blob.w() / blob.h() > 1 - rect_threshold and blob.w() / blob.h() < 1 + rect_threshold:
uart.write("Blue rectangle\n")
# 形状检测:圆形
elif blob.circularity() > circle_threshold:
uart.write("Blue circle\n")
# 形状检测:五角星
elif blob.corners() == 5 and blob.area() > 500 and blob.area() < 5000:
uart.write("Blue star\n")
# 形状检测:三角形
elif blob.corners() == 3 and blob.area() > 500 and blob.area() < 5000:
uart.write("Blue triangle\n")
# 识别绿色
blobs = img.find_blobs([green_threshold])
for blob in blobs:
# 形状检测:长方形
if blob.w() / blob.h() > 1 - rect_threshold and blob.w() / blob.h() < 1 + rect_threshold:
uart.write("Green rectangle\n")
# 形状检测:圆形
elif blob.circularity() > circle_threshold:
uart.write("Green circle\n")
# 形状检测:五角星
elif blob.corners() == 5 and blob.area() > 500 and blob.area() < 5000:
uart.write("Green star\n")
# 形状检测:三角形
elif blob.corners() == 3 and blob.area() > 500 and blob.area() < 5000:
uart.write("Green triangle\n")
```
这个代码使用`find_blobs()`函数识别颜色,使用`circularity()`和`corners()`函数进行形状检测。其中,`rect_threshold`、`circle_threshold`、`star_threshold`和`triangle_threshold`分别表示长方形、圆形、五角星和三角形的形状阈值,可以根据实际情况进行调整。`uart.write()`函数用于将识别结果发送到串口。
相关推荐
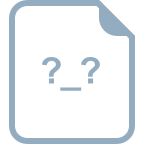
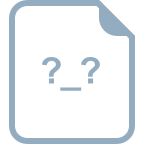














