编写一个程序,根据从标准输入接收的指令来维护和操作排序的链表。链表是按顺 序维护的,这意味着链表中的数据在每次操作后都以递增的数字顺序存储。 请注意,在创建新节点时,需要使用malloc为它们分配空间;一旦不再需要任何已 分配的空间,就应该使用free将其释放。还要注意,链表不包含重复的值。 2.2【基本要求】 链表支持两种操作指令。 插入n:向链表中添加一个整数n。如果链表中已经存在n,则它什么也不做。指令 格式是一个i后跟一个空格和一个整数n。 删除n:从链表中删除一个整数n。如果链表中不存在n,它什么也不做。指令格式 是d后跟一个空格和一个整数n。 在每个命令之后,程序将输出链表的长度,然后是链表的内容,按照从第一个(最 小)到最后一个(最大)的顺序。 输入格式:输入的每一行都包含一条指令。每行都以一个字母(或者是“i”或者是 “d”)开头,后跟一个空格,然后是一个整数。以“i”开头的一行表示该整数应该插 入链表中。以“d”开头的一行表示应从链表中删除该整数。输入i和d以外的字符程序 结束运行。 输出格式:执行每个指令后,程序将输出一行文本,其中包含链表的长度、一个冒 号以及按顺序排列的链表元素,所有内容都用空格分隔。 程序运行操作示例:(i或d开头的行是输入行,其他是输出行) i 5 1: 5 d 3 1: 5 i 3 2: 3 5 i 500 3: 3 5 500 d 5 2: 3 500
时间: 2024-03-26 08:42:28 浏览: 63
以下是一个C语言的实现,注意需要手动输入结束符号才能结束程序:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int val;
struct Node *next;
} Node;
Node *head = NULL;
void insert(int n) {
Node *newNode = (Node*) malloc(sizeof(Node));
newNode->val = n;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
printf("1: %d\n", n);
return;
}
if (n < head->val) {
newNode->next = head;
head = newNode;
printf("%d: ", count());
printList();
return;
}
Node *current = head;
while (current->next != NULL && current->next->val < n) {
current = current->next;
}
if (current->val == n) {
printf("%d: ", count());
printList();
return;
}
if (current->next != NULL && current->next->val == n) {
printf("%d: ", count());
printList();
return;
}
newNode->next = current->next;
current->next = newNode;
printf("%d: ", count());
printList();
}
void delete(int n) {
if (head == NULL) {
printf("0: \n");
return;
}
if (head->val == n) {
Node *temp = head;
head = head->next;
free(temp);
printf("%d: ", count());
printList();
return;
}
Node *current = head;
while (current->next != NULL && current->next->val < n) {
current = current->next;
}
if (current->next == NULL || current->next->val != n) {
printf("%d: ", count());
printList();
return;
}
Node *temp = current->next;
current->next = current->next->next;
free(temp);
printf("%d: ", count());
printList();
}
int count() {
int count = 0;
Node *current = head;
while (current != NULL) {
count++;
current = current->next;
}
return count;
}
void printList() {
Node *current = head;
while (current != NULL) {
printf("%d ", current->val);
current = current->next;
}
printf("\n");
}
int main() {
char cmd;
int n;
while (1) {
scanf("%c %d", &cmd, &n);
if (cmd == 'i') {
insert(n);
} else if (cmd == 'd') {
delete(n);
} else {
break;
}
getchar(); // 读取并忽略回车符
}
return 0;
}
```
阅读全文
相关推荐
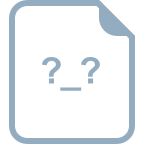
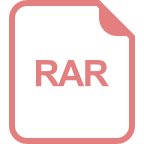


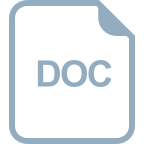
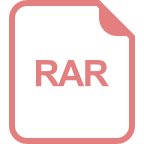
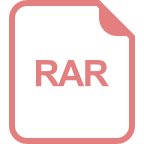
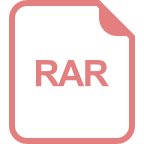
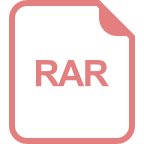
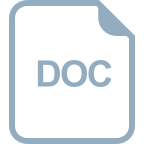
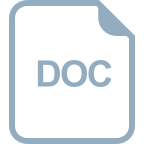
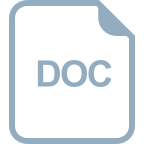
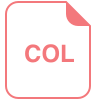
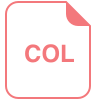
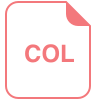
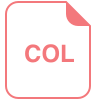
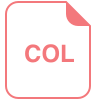