BuildAdmin vue3 formitem 添加Cascader
时间: 2023-07-12 07:05:07 浏览: 91
在 BuildAdmin 框架中,可以通过在 `formitem` 中添加 `Cascader` 组件来实现级联选择器的功能。在 Vue 3 中,可以使用 `defineComponent` 和 `ref` 来定义组件,并使用 `setup` 函数来编写逻辑代码。
具体实现步骤如下:
1. 在 `setup` 函数中定义 `area` 和 `options` 变量,并通过 `ref` 创建响应式引用。
```vue
<template>
<a-form-item label="所在地区">
<a-cascader v-model="area" :options="options" />
</a-form-item>
</template>
<script>
import { defineComponent, ref } from 'vue'
export default defineComponent({
setup() {
const area = ref([])
const options = ref([
{
value: 'zhejiang',
label: '浙江',
children: [
{
value: 'hangzhou',
label: '杭州',
children: [
{
value: 'xihu',
label: '西湖'
}
]
}
]
},
{
value: 'jiangsu',
label: '江苏',
children: [
{
value: 'nanjing',
label: '南京',
children: [
{
value: 'zhonghuamen',
label: '中华门'
}
]
}
]
}
])
return {
area,
options
}
}
})
</script>
```
2. 在 `loadData` 方法中,使用异步请求从服务器获取子选项列表,并将其赋值给 `children` 属性。在 Vue 3 中,可以使用 `async/await` 来简化异步操作。
```vue
<template>
<a-form-item label="所在地区">
<a-cascader v-model="area" :options="options" :load-data="loadData" />
</a-form-item>
</template>
<script>
import { defineComponent, ref } from 'vue'
export default defineComponent({
setup() {
const area = ref([])
const options = ref([])
const loadData = async (selectedOptions) => {
const targetOption = selectedOptions[selectedOptions.length - 1]
targetOption.loading = true
const data = await fetch(`https://api.example.com/${targetOption.value}`)
targetOption.children = data
targetOption.loading = false
}
return {
area,
options,
loadData
}
}
})
</script>
```
注意:在使用级联选择器时,需要注意选项列表的数据格式和选项的 `value` 属性。在示例代码中,选项的 `value` 属性为字符串类型,如果需要使用其他类型的值,需要根据实际情况进行调整。同时,需要注意在 `loadData` 方法中修改属性时,需要使用 `targetOption` 变量来引用选项对象,否则会导致响应式失效。
阅读全文
相关推荐
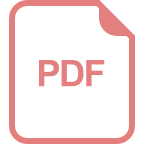
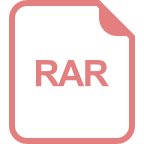

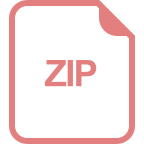
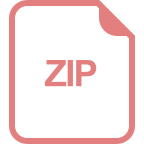
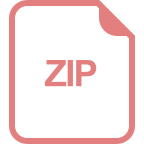
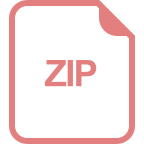
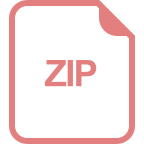
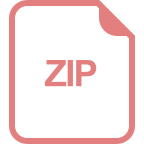
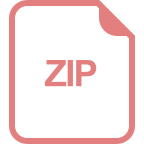
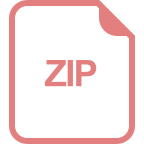
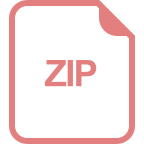
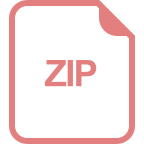
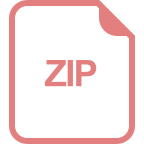
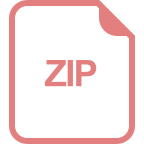
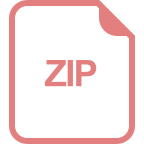