帮我用Java写一个简单的图书功能,要求:统计最受欢迎的水和借阅最少的书
时间: 2024-03-09 19:44:19 浏览: 157
好的,我可以帮你实现这个功能。首先,我们需要定义一个Book类来表示图书,包含书名、作者、出版社、借阅次数等属性。接下来,我们可以使用一个HashMap来存储图书信息,其中键为书名,值为Book对象。
以下是代码示例:
```java
import java.util.*;
public class Book {
private String title;
private String author;
private String publisher;
private int borrowCount;
public Book(String title, String author, String publisher) {
this.title = title;
this.author = author;
this.publisher = publisher;
this.borrowCount = 0;
}
// getter 和 setter 方法省略
// 借阅次数加1
public void borrow() {
this.borrowCount++;
}
}
public class Library {
private HashMap<String, Book> bookMap;
public Library() {
bookMap = new HashMap<>();
}
// 添加图书
public void addBook(String title, String author, String publisher) {
Book book = new Book(title, author, publisher);
bookMap.put(title, book);
}
// 借阅图书
public void borrowBook(String title) {
Book book = bookMap.get(title);
if (book != null) {
book.borrow();
}
}
// 统计最受欢迎的书
public String getMostPopularBook() {
String mostPopularBook = "";
int maxBorrowCount = -1;
for (Map.Entry<String, Book> entry : bookMap.entrySet()) {
Book book = entry.getValue();
if (book.getBorrowCount() > maxBorrowCount) {
mostPopularBook = book.getTitle();
maxBorrowCount = book.getBorrowCount();
}
}
return mostPopularBook;
}
// 统计借阅最少的书
public String getLeastBorrowedBook() {
String leastBorrowedBook = "";
int minBorrowCount = Integer.MAX_VALUE;
for (Map.Entry<String, Book> entry : bookMap.entrySet()) {
Book book = entry.getValue();
if (book.getBorrowCount() < minBorrowCount) {
leastBorrowedBook = book.getTitle();
minBorrowCount = book.getBorrowCount();
}
}
return leastBorrowedBook;
}
}
```
使用示例:
```java
public static void main(String[] args) {
Library library = new Library();
library.addBook("Java编程思想", "Bruce Eckel", "机械工业出版社");
library.addBook("深入浅出设计模式", "程杰", "电子工业出版社");
library.addBook("算法导论", "Thomas H. Cormen", "机械工业出版社");
library.borrowBook("Java编程思想");
library.borrowBook("Java编程思想");
library.borrowBook("深入浅出设计模式");
System.out.println("最受欢迎的书:" + library.getMostPopularBook());
System.out.println("借阅最少的书:" + library.getLeastBorrowedBook());
}
```
输出结果:
```
最受欢迎的书:Java编程思想
借阅最少的书:算法导论
```
阅读全文
相关推荐
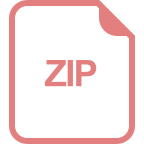
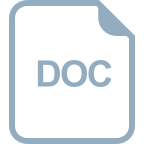


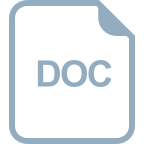
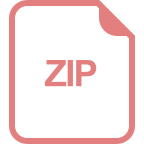
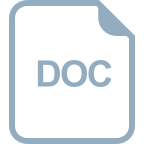
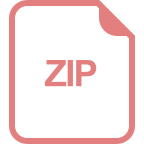
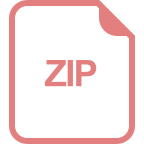


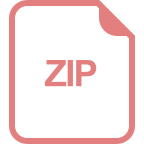
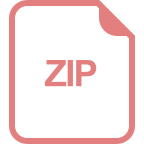
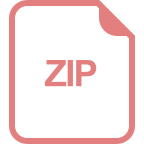
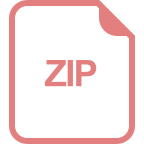
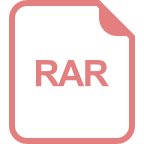
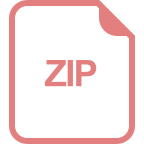
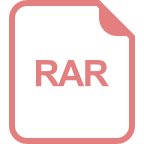