java 将一个类的构造方法变为单例
时间: 2024-01-05 20:10:27 浏览: 61
可以通过将类的构造方法私有化,同时提供一个静态方法来获取该类的唯一实例来实现将一个类的构造方法变为单例。以下是一个示例代码:
```
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
```
在上面的例子中,构造方法被私有化,所以外部无法通过 new 关键字创建该类的实例。同时提供了一个静态的 getInstance() 方法,通过该方法获取 Singleton 类的唯一实例。在该方法中,如果 instance 为 null,则创建一个新的 Singleton 实例,否则返回已有的实例。这样就可以保证 Singleton 类只有一个实例存在。
相关问题
、选一种方法把玩家的飞机(不是敌机)变成单例类(上传代码)
在Java中,我们可以使用Singleton模式来创建一个全局唯一的玩家飞机实例。以下是通过构造函数锁定和静态内部类实现的简单示例:
```java
public class PlayerPlane {
// 私有化构造函数,防止外部直接创建实例
private PlayerPlane() {}
// 全局唯一实例
private static volatile PlayerPlane instance;
// 获取单例方法
public static PlayerPlane getInstance() {
if (instance == null) {
synchronized (PlayerPlane.class) {
if (instance == null) {
instance = new PlayerPlane();
}
}
}
return instance;
}
// 其他方法,如飞机的行为和属性...
public void move() {
// 飞机移动的逻辑...
}
public void fire() {
// 发射导弹的逻辑...
}
}
```
在这个例子中,`getInstance()`方法负责创建并返回单例。通过`volatile`关键字保证了线程安全,即使在多线程环境中也能确保只有一个实例。
阅读全文
相关推荐
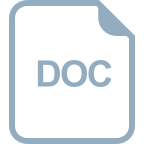
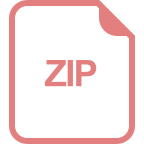
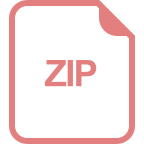
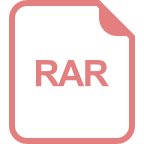
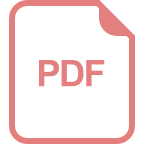
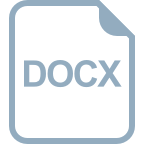
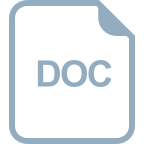
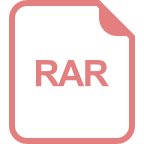
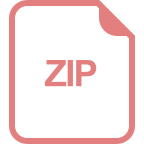
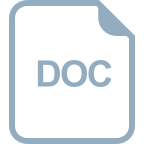
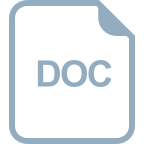
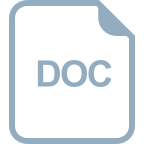
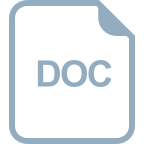
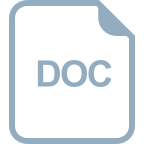