springboot实现网页端增删改查代码
时间: 2023-06-17 08:02:00 浏览: 61
这里提供一个简单的示例,使用Spring Boot和Thymeleaf模板引擎实现网页端的增删改查功能。
首先,创建一个实体类(例如Student),包含需要存储的属性,如id、name、age等。
```java
@Entity
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Integer age;
// 省略getter和setter
}
```
接下来,创建一个Repository接口,继承自JpaRepository,用于实现对数据库的增删改查操作。
```java
public interface StudentRepository extends JpaRepository<Student, Long> {
}
```
然后,创建一个控制器类,用于处理请求和响应。在控制器中,注入StudentRepository,用于处理对数据库的操作。
```java
@Controller
public class StudentController {
@Autowired
private StudentRepository studentRepository;
@RequestMapping("/")
public String index(Model model) {
List<Student> students = studentRepository.findAll();
model.addAttribute("students", students);
return "index";
}
@GetMapping("/add")
public String addForm(Model model) {
model.addAttribute("student", new Student());
return "add";
}
@PostMapping("/add")
public String addSubmit(@ModelAttribute Student student) {
studentRepository.save(student);
return "redirect:/";
}
@GetMapping("/edit/{id}")
public String editForm(@PathVariable Long id, Model model) {
Student student = studentRepository.findById(id)
.orElseThrow(() -> new IllegalArgumentException("Invalid student Id:" + id));
model.addAttribute("student", student);
return "edit";
}
@PostMapping("/edit/{id}")
public String editSubmit(@PathVariable Long id, @ModelAttribute Student student) {
student.setId(id);
studentRepository.save(student);
return "redirect:/";
}
@GetMapping("/delete/{id}")
public String delete(@PathVariable Long id) {
studentRepository.deleteById(id);
return "redirect:/";
}
}
```
在控制器中,定义了以下请求处理方法:
- index:用于显示所有学生数据的页面;
- addForm:用于显示添加学生数据的页面;
- addSubmit:用于处理添加学生数据的请求;
- editForm:用于显示修改学生数据的页面;
- editSubmit:用于处理修改学生数据的请求;
- delete:用于处理删除学生数据的请求。
最后,创建Thymeleaf模板,用于显示网页内容。在模板中,使用Thymeleaf提供的语法,实现对控制器中注入的数据进行展示和操作。
例如,index.html模板的代码如下:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>学生信息</title>
</head>
<body>
<h1>学生信息</h1>
<table>
<thead>
<tr>
<th>编号</th>
<th>姓名</th>
<th>年龄</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr th:each="student : ${students}">
<td th:text="${student.id}"></td>
<td th:text="${student.name}"></td>
<td th:text="${student.age}"></td>
<td>
<a th:href="@{/edit/{id}(id=${student.id})}">编辑</a>
<a th:href="@{/delete/{id}(id=${student.id})}">删除</a>
</td>
</tr>
</tbody>
</table>
<a href="/add">添加学生信息</a>
</body>
</html>
```
以上示例代码中,使用Thymeleaf语法实现了对学生信息进行展示和操作,并且通过控制器中的请求处理方法,实现了对数据库的增删改查操作。
需要注意的是,示例代码中省略了异常处理、表单校验、安全性等方面的处理,实际开发中需要根据具体需求进行实现。
相关推荐
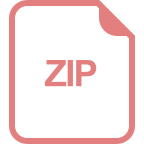














