筛选车位检测结果中的冗余车位线pyhon代码
时间: 2023-06-26 21:03:24 浏览: 49
这里提供一种基于霍夫变换的方法来筛选车位检测结果中的冗余车位线。假设已经得到了车位检测的所有直线,以下是基于霍夫变换的筛选代码:
```python
import numpy as np
import cv2
# 假设lines为所有检测到的车位直线,threshold为筛选阈值
def filter_redundant_lines(lines, threshold):
# 对直线按照极角进行聚类,得到每个簇中的直线
angles = [np.arctan2(line[1]-line[3], line[0]-line[2]) for line in lines]
clusters = {}
for i, angle in enumerate(angles):
if angle not in clusters:
clusters[angle] = []
clusters[angle].append(lines[i])
# 对每个簇中的直线按照长度排序,取最长的直线作为代表
representatives = []
for angle, group in clusters.items():
group = sorted(group, key=lambda x: np.abs(x[0]-x[2])+np.abs(x[1]-x[3]), reverse=True)
representatives.append(group[0])
# 计算每条直线与其他代表直线之间的距离
distances = np.zeros((len(representatives), len(representatives)))
for i in range(len(representatives)):
for j in range(i+1, len(representatives)):
p1, p2 = representatives[i][:2], representatives[i][2:]
q1, q2 = representatives[j][:2], representatives[j][2:]
dist = np.abs(np.cross(p2-p1, q1-p1)) / np.linalg.norm(p2-p1)
distances[i][j] = distances[j][i] = dist
# 根据距离矩阵,计算每个代表直线是否与其他直线重叠
overlaps = np.zeros((len(representatives), len(representatives)))
for i in range(len(representatives)):
for j in range(i+1, len(representatives)):
if distances[i][j] < threshold:
overlaps[i][j] = overlaps[j][i] = 1
# 将重叠的直线合并成一个直线,并去除冗余直线
keep = [True]*len(representatives)
for i in range(len(representatives)):
for j in range(i+1, len(representatives)):
if overlaps[i][j]:
keep[i] = False
break
if not keep[i]:
continue
for j in range(i+1, len(representatives)):
if overlaps[i][j]:
p1, p2 = representatives[i][:2], representatives[i][2:]
q1, q2 = representatives[j][:2], representatives[j][2:]
new_line = np.array([p1, p2, q1, q2], dtype=np.int32)
length1 = np.abs(p1[0]-p2[0])+np.abs(p1[1]-p2[1])
length2 = np.abs(q1[0]-q2[0])+np.abs(q1[1]-q2[1])
if length1 > length2:
representatives[j] = new_line
else:
representatives[i] = new_line
keep[j] = False
# 返回最终的直线列表
result = []
for i in range(len(representatives)):
if keep[i]:
result.append(representatives[i])
return result
```
这个函数将返回一个筛选后的车位直线列表,可以直接用于后续车位标记的工作中。注意,这个函数并不适用于所有情况,因为不同的车场、摄像头、光照条件下得到的车位检测结果可能差异很大。因此,在实际应用中,需要根据具体情况进行调整和优化。
相关推荐
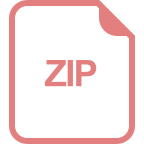
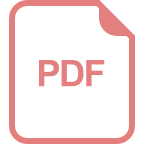
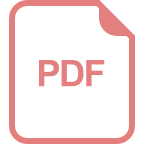














