学生成绩管理系统c语言代码详细数据结构设计
时间: 2023-07-31 16:09:39 浏览: 112
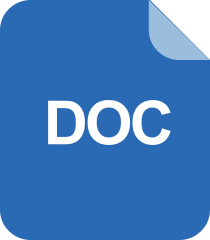
c语言学生成绩管理系统代码
学生成绩管理系统的C语言代码涉及到以下几个方面的数据结构设计:
1. 学生信息结构体
学生信息结构体包含学生的学号、姓名、性别、年龄、班级等信息,用来存储每个学生的个人信息。
```c
typedef struct{
char id[20]; // 学号
char name[20]; // 姓名
char sex[5]; // 性别
int age; // 年龄
char class[20]; // 班级
} Student;
```
2. 成绩信息结构体
成绩信息结构体包含学生的学号、课程名、成绩等信息,用来存储每个学生的成绩信息。
```c
typedef struct{
char id[20]; // 学号
char course[20]; // 课程名
float score; // 成绩
} Score;
```
3. 链表节点结构体
链表节点结构体用来实现链表数据结构,包含指向下一个节点的指针和节点数据。
```c
typedef struct node{
void* data; // 节点数据指针
struct node* next; // 指向下一个节点的指针
} Node;
```
4. 链表结构体
链表结构体用来实现链表数据结构,包含链表的头节点指针和链表的长度信息。
```c
typedef struct{
Node* head; // 链表头节点指针
int length; // 链表长度
} LinkedList;
```
以上是学生成绩管理系统C语言代码的数据结构设计,可以根据实际需要进行调整和扩展。具体的代码实现可以参考以下示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 学生信息结构体
typedef struct{
char id[20]; // 学号
char name[20]; // 姓名
char sex[5]; // 性别
int age; // 年龄
char class[20]; // 班级
} Student;
// 成绩信息结构体
typedef struct{
char id[20]; // 学号
char course[20]; // 课程名
float score; // 成绩
} Score;
// 链表节点结构体
typedef struct node{
void* data; // 节点数据指针
struct node* next; // 指向下一个节点的指针
} Node;
// 链表结构体
typedef struct{
Node* head; // 链表头节点指针
int length; // 链表长度
} LinkedList;
// 初始化链表
LinkedList* initLinkedList(){
LinkedList* list = (LinkedList*)malloc(sizeof(LinkedList));
list->head = NULL;
list->length = 0;
return list;
}
// 创建节点
Node* createNode(void* data){
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
return node;
}
// 链表头部插入节点
void insertHead(LinkedList* list, void* data){
Node* node = createNode(data);
node->next = list->head;
list->head = node;
list->length++;
}
// 链表尾部插入节点
void insertTail(LinkedList* list, void* data){
Node* node = createNode(data);
if(list->head == NULL){
list->head = node;
}else{
Node* p = list->head;
while(p->next != NULL){
p = p->next;
}
p->next = node;
}
list->length++;
}
// 遍历链表
void traverseLinkedList(LinkedList* list, void (*visit)(void*)){
Node* p = list->head;
while(p != NULL){
visit(p->data);
p = p->next;
}
}
// 打印学生信息
void printStudent(void* data){
Student* student = (Student*)data;
printf("ID: %s, Name: %s, Sex: %s, Age: %d, Class: %s\n", student->id, student->name, student->sex, student->age, student->class);
}
// 打印成绩信息
void printScore(void* data){
Score* score = (Score*)data;
printf("ID: %s, Course: %s, Score: %.2f\n", score->id, score->course, score->score);
}
// 释放节点
void freeNode(Node* node){
free(node->data);
free(node);
}
// 释放链表
void freeLinkedList(LinkedList* list){
Node* p = list->head;
while(p != NULL){
Node* q = p->next;
freeNode(p);
p = q;
}
free(list);
}
// 添加学生信息
void addStudent(LinkedList* list){
Student* student = (Student*)malloc(sizeof(Student));
printf("Please input student ID: ");
scanf("%s", student->id);
printf("Please input student name: ");
scanf("%s", student->name);
printf("Please input student sex: ");
scanf("%s", student->sex);
printf("Please input student age: ");
scanf("%d", &student->age);
printf("Please input student class: ");
scanf("%s", student->class);
insertTail(list, student);
printf("Add student success.\n");
}
// 添加成绩信息
void addScore(LinkedList* list){
Score* score = (Score*)malloc(sizeof(Score));
printf("Please input student ID: ");
scanf("%s", score->id);
printf("Please input course name: ");
scanf("%s", score->course);
printf("Please input score: ");
scanf("%f", &score->score);
insertTail(list, score);
printf("Add score success.\n");
}
// 查询学生信息
void queryStudent(LinkedList* list){
char id[20];
printf("Please input student ID: ");
scanf("%s", id);
Node* p = list->head;
while(p != NULL){
Student* student = (Student*)p->data;
if(strcmp(student->id, id) == 0){
printStudent(student);
return;
}
p = p->next;
}
printf("Student not found.\n");
}
// 查询成绩信息
void queryScore(LinkedList* list){
char id[20];
printf("Please input student ID: ");
scanf("%s", id);
Node* p = list->head;
while(p != NULL){
Score* score = (Score*)p->data;
if(strcmp(score->id, id) == 0){
printScore(score);
}
p = p->next;
}
}
// 删除学生信息
void deleteStudent(LinkedList* list){
char id[20];
printf("Please input student ID: ");
scanf("%s", id);
Node* p = list->head;
Node* pre = NULL;
while(p != NULL){
Student* student = (Student*)p->data;
if(strcmp(student->id, id) == 0){
if(pre == NULL){
list->head = p->next;
}else{
pre->next = p->next;
}
freeNode(p);
list->length--;
printf("Delete student success.\n");
return;
}
pre = p;
p = p->next;
}
printf("Student not found.\n");
}
// 删除成绩信息
void deleteScore(LinkedList* list){
char id[20];
char course[20];
printf("Please input student ID: ");
scanf("%s", id);
printf("Please input course name: ");
scanf("%s", course);
Node* p = list->head;
Node* pre = NULL;
while(p != NULL){
Score* score = (Score*)p->data;
if(strcmp(score->id, id) == 0 && strcmp(score->course, course) == 0){
if(pre == NULL){
list->head = p->next;
}else{
pre->next = p->next;
}
freeNode(p);
list->length--;
printf("Delete score success.\n");
return;
}
pre = p;
p = p->next;
}
printf("Score not found.\n");
}
// 显示菜单
void showMenu(){
printf("1. Add student information\n");
printf("2. Add score information\n");
printf("3. Query student information\n");
printf("4. Query score information\n");
printf("5. Delete student information\n");
printf("6. Delete score information\n");
printf("7. Exit\n");
}
int main(){
LinkedList* list = initLinkedList();
int choice = 0;
while(1){
showMenu();
printf("Please select a function: ");
scanf("%d", &choice);
switch(choice){
case 1:
addStudent(list);
break;
case 2:
addScore(list);
break;
case 3:
queryStudent(list);
break;
case 4:
queryScore(list);
break;
case 5:
deleteStudent(list);
break;
case 6:
deleteScore(list);
break;
case 7:
freeLinkedList(list);
printf("Exit.\n");
return 0;
default:
printf("Invalid input.\n");
}
}
return 0;
}
```
阅读全文
相关推荐
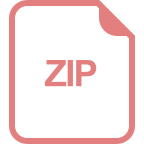

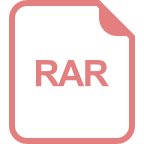
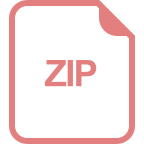
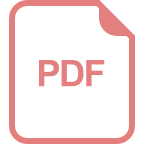
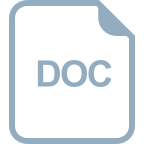
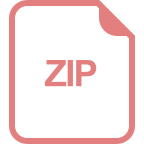
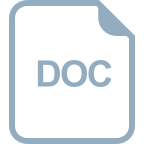
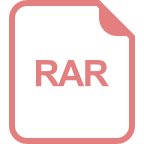
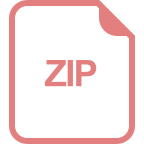
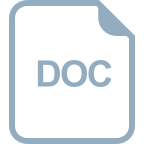
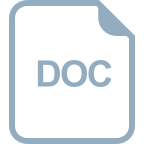

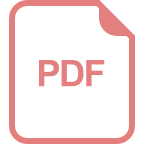