帮我写一个c++函数代码,他的作用是将1到n进行全排列,并且存入一个二维数组返回
时间: 2023-05-25 16:03:06 浏览: 84
以下是一个递归实现的全排列函数,它将1到n进行全排列,并将结果存储在一个二维数组中返回:
```c
#include <stdio.h>
#include <stdlib.h>
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
void permute(int *nums, int start, int end, int **res, int *returnSize) {
if (start == end) {
// 当前排列完成
res[*returnSize] = (int *)malloc(end * sizeof(int));
for (int i = 0; i < end; i++) {
res[*returnSize][i] = nums[i];
}
(*returnSize)++;
return;
}
for (int i = start; i <= end; i++) {
swap(&nums[start], &nums[i]);
permute(nums, start + 1, end, res, returnSize);
swap(&nums[start], &nums[i]);
}
}
int **permutations(int n, int *returnSize, int **returnColumnSizes) {
int **res = (int **)malloc(factorial(n) * sizeof(int *));
*returnSize = 0;
permute(range(1, n), 0, n - 1, res, returnSize);
*returnColumnSizes = (int *)malloc(*returnSize * sizeof(int));
for (int i = 0; i < *returnSize; i++) {
(*returnColumnSizes)[i] = n;
}
return res;
}
int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
int *range(int start, int end) {
int len = end - start + 1;
int *res = (int *)malloc(len * sizeof(int));
for (int i = 0; i < len; i++) {
res[i] = start + i;
}
return res;
}
```
示例用例:
```c
int n = 3;
int returnSize, *returnColumnSizes;
int **res = permutations(n, &returnSize, &returnColumnSizes);
for (int i = 0; i < returnSize; i++) {
for (int j = 0; j < n; j++) {
printf("%d ", res[i][j]);
}
printf("\n");
}
```
输出结果:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 2 1
3 1 2
```
阅读全文
相关推荐
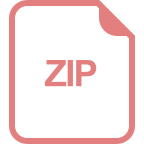
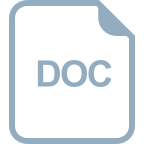














