要求用户输入一个问题和一段材料,用python语言实现在给定材料中抽取问题答案的功能
时间: 2024-05-01 18:21:18 浏览: 133
可以使用自然语言处理库spaCy来实现这个功能。首先需要安装spaCy库和其英文语言模型。
```python
!pip install spacy
!python -m spacy download en_core_web_sm
```
接下来,可以使用以下代码来实现在给定材料中抽取问题答案的功能。
```python
import spacy
nlp = spacy.load("en_core_web_sm")
def extract_answer(question, passage):
question_doc = nlp(question)
passage_doc = nlp(passage)
# 找到与问题最相关的句子
sentences = [sent.text.strip() for sent in passage_doc.sents]
sentence_scores = []
for sentence in sentences:
sentence_doc = nlp(sentence)
score = question_doc.similarity(sentence_doc)
sentence_scores.append(score)
best_sentence = sentences[sentence_scores.index(max(sentence_scores))]
# 找到最相关句子中的答案
best_sentence_doc = nlp(best_sentence)
answer = ""
for ent in best_sentence_doc.ents:
if ent.label_ in ["DATE", "TIME", "PERSON", "NORP", "ORG", "GPE", "LOC", "PRODUCT", "EVENT", "WORK_OF_ART"]:
answer = ent.text
break
if answer:
return answer
else:
for token in best_sentence_doc:
if token.pos_ in ["NOUN", "PROPN"] and not token.is_stop:
answer = token.text
break
return answer
```
这个函数接受两个参数:问题和材料。它首先使用spaCy库将它们转换为文档对象。然后它将材料中的每个句子与问题进行比较,并找到最相关的句子。最后,它在最相关的句子中查找实体或名词作为答案,并将其返回。如果无法找到答案,则返回空字符串。
接下来可以测试一下这个函数。
```python
question = "What is the capital of France?"
passage = "France is a country located in Western Europe. Its capital is Paris and its currency is the Euro."
answer = extract_answer(question, passage)
print(answer) # 输出:Paris
```
可以看到,函数成功地从材料中提取出了问题的答案。
阅读全文
相关推荐
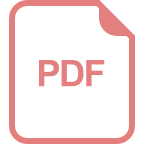
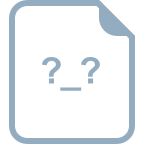
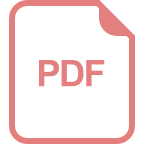
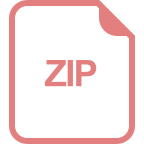
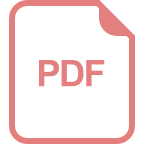
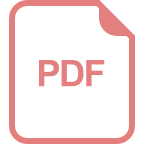
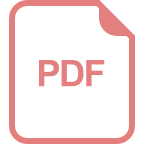
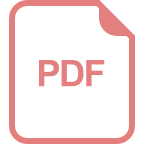
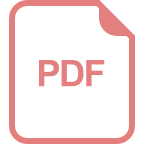
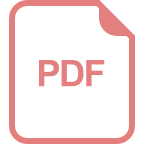
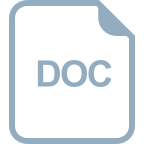
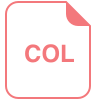
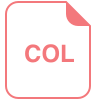
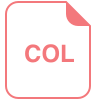
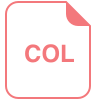
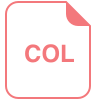
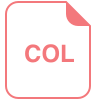
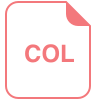
