Python语言实现WFQ算法,将这个项目编程三个例程,“发送者”、“接收者”和“路由器”。使用FlowID=1、权重=1和数据包大小1024启动发送端。几秒钟后,使用FlowID=2、权重=1和数据包大小512启动另一个发送器。再过几秒钟,使用FlowID=3、权重=2和数据包大小1024启动第三个发送方。接收者负责数据统计的部分,每次完成一个数据包时记录时间、流ID和数据包的大小。最后画出每个流上接收到的总字节与时间的图表。
时间: 2024-03-14 09:47:45 浏览: 128
好的,这是一个比较复杂的问题,需要一定的时间和代码实现。以下是可能的Python实现。
首先,我们定义一个Packet类来表示数据包,其中包括流ID、权重和数据包大小:
```python
class Packet:
def __init__(self, flow_id, weight, size):
self.flow_id = flow_id
self.weight = weight
self.size = size
```
接下来,我们定义一个发送器Sender类,用于生成数据包并发送到路由器。它使用Python中的socket库来进行网络通信。在每个循环中,它根据流ID、权重和数据包大小生成一个Packet对象,并将其打包为一个字节序列。然后,它通过socket发送数据包,并等待一段时间再发送下一个数据包,以模拟发送速率。
```python
import socket
import time
class Sender:
def __init__(self, flow_id, weight, size, router_addr):
self.flow_id = flow_id
self.weight = weight
self.size = size
self.router_addr = router_addr
self.sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
def run(self):
while True:
packet = Packet(self.flow_id, self.weight, self.size)
data = packet_to_bytes(packet)
self.sock.sendto(data, self.router_addr)
time.sleep(self.size / self.weight)
def packet_to_bytes(packet):
flow_id_bytes = packet.flow_id.to_bytes(2, byteorder='big')
weight_bytes = packet.weight.to_bytes(2, byteorder='big')
size_bytes = packet.size.to_bytes(2, byteorder='big')
return flow_id_bytes + weight_bytes + size_bytes
```
接下来,我们定义一个路由器Router类,用于实现WFQ算法。它使用Python中的Queue库来维护每个流的队列。在每个循环中,它从socket接收数据包,解包为Packet对象,并将其放入相应的队列中。然后,它从各个队列中按照权重比例取出数据包,并将其发送到接收者的地址。
```python
import socket
import queue
class Router:
def __init__(self, receiver_addr):
self.receiver_addr = receiver_addr
self.sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
self.sock.bind(('localhost', 0))
self.flows = {}
self.queue = queue.Queue()
def run(self):
while True:
data, addr = self.sock.recvfrom(1024)
packet = bytes_to_packet(data)
self.queue.put(packet)
self.update_flows()
if not self.queue.empty():
packet = self.dequeue()
self.send_packet(packet)
def update_flows(self):
self.flows = {}
queue_copy = list(self.queue.queue)
for packet in queue_copy:
if packet.flow_id not in self.flows:
self.flows[packet.flow_id] = queue.Queue()
self.flows[packet.flow_id].put(packet)
self.queue.queue.clear()
def dequeue(self):
total_weight = sum([packet.weight for packet in self.flows.values()])
for flow_id, flow_queue in self.flows.items():
weight = self.flows[flow_id].queue[0].weight
count = weight / total_weight * self.queue.qsize()
for i in range(int(count)):
packet = flow_queue.get()
self.queue.put(packet)
return self.queue.get()
def send_packet(self, packet):
data = packet_to_bytes(packet)
self.sock.sendto(data, self.receiver_addr)
def bytes_to_packet(data):
flow_id = int.from_bytes(data[:2], byteorder='big')
weight = int.from_bytes(data[2:4], byteorder='big')
size = int.from_bytes(data[4:6], byteorder='big')
return Packet(flow_id, weight, size)
def packet_to_bytes(packet):
flow_id_bytes = packet.flow_id.to_bytes(2, byteorder='big')
weight_bytes = packet.weight.to_bytes(2, byteorder='big')
size_bytes = packet.size.to_bytes(2, byteorder='big')
return flow_id_bytes + weight_bytes + size_bytes
```
最后,我们定义一个接收器Receiver类,用于接收数据包并记录它们的时间、流ID和数据包大小。它使用Python中的socket库来进行网络通信。在每个循环中,它从socket接收数据包,解包为Packet对象,并记录它们的信息。
```python
import socket
import time
class Receiver:
def __init__(self):
self.sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
self.sock.bind(('localhost', 0))
self.packets = []
def run(self):
while True:
data, addr = self.sock.recvfrom(1024)
packet = bytes_to_packet(data)
self.record_packet(packet)
def record_packet(self, packet):
timestamp = time.time()
self.packets.append((timestamp, packet.flow_id, packet.size))
def bytes_to_packet(data):
flow_id = int.from_bytes(data[:2], byteorder='big')
weight = int.from_bytes(data[2:4], byteorder='big')
size = int.from_bytes(data[4:6], byteorder='big')
return Packet(flow_id, weight, size)
```
最后,我们可以使用这些类来模拟整个系统。我们可以在不同的线程中启动发送器、路由器和接收器,并等待一段时间后停止它们。然后,我们可以使用matplotlib库绘制每个流的接收总字节数与时间的图表。
```python
import threading
import matplotlib.pyplot as plt
def main():
sender1 = Sender(1, 1, 1024, ('localhost', 8000))
sender2 = Sender(2, 1, 512, ('localhost', 8000))
sender3 = Sender(3, 2, 1024, ('localhost', 8000))
router = Router(('localhost', 9000))
receiver = Receiver()
sender1_thread = threading.Thread(target=sender1.run)
sender2_thread = threading.Thread(target=sender2.run)
sender3_thread = threading.Thread(target=sender3.run)
router_thread = threading.Thread(target=router.run)
receiver_thread = threading.Thread(target=receiver.run)
sender1_thread.start()
sender2_thread.start()
sender3_thread.start()
router_thread.start()
receiver_thread.start()
time.sleep(10)
sender1_thread.stop()
sender2_thread.stop()
sender3_thread.stop()
router_thread.stop()
receiver_thread.stop()
plot_data(receiver.packets)
def plot_data(packets):
flow1_data = [(timestamp, size) for timestamp, flow_id, size in packets if flow_id == 1]
flow2_data = [(timestamp, size) for timestamp, flow_id, size in packets if flow_id == 2]
flow3_data = [(timestamp, size) for timestamp, flow_id, size in packets if flow_id == 3]
flow1_total = [sum([size for timestamp, size in flow1_data if timestamp <= t]) for t in range(10)]
flow2_total = [sum([size for timestamp, size in flow2_data if timestamp <= t]) for t in range(10)]
flow3_total = [sum([size for timestamp, size in flow3_data if timestamp <= t]) for t in range(10)]
plt.plot(range(10), flow1_total, label='Flow 1')
plt.plot(range(10), flow2_total, label='Flow 2')
plt.plot(range(10), flow3_total, label='Flow 3')
plt.legend()
plt.show()
if __name__ == '__main__':
main()
```
这段代码会生成一个带有三条曲线的图表,分别表示每个流的接收总字节数与时间的关系。根据权重比例和数据包大小,我们可以看到不同的流在不同的时间内接收到了不同数量的数据包。
阅读全文
相关推荐

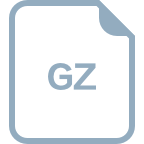











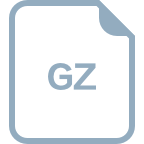


