qt 怎么在scene上绘制矩形
时间: 2024-02-22 11:54:48 浏览: 27
要在Qt的场景(Scene)上绘制一个矩形,你可以使用QGraphicsRectItem类。以下是在场景上绘制矩形的基本步骤:
1. 创建QGraphicsRectItem对象,并设置其大小和位置:
```
QGraphicsRectItem *rect = new QGraphicsRectItem(x, y, width, height);
```
其中,x和y是矩形左上角的坐标,width和height是矩形的宽度和高度。
2. 将矩形添加到场景中:
```
QGraphicsScene *scene = new QGraphicsScene();
scene->addItem(rect);
```
3. 在场景视图中显示场景:
```
QGraphicsView *view = new QGraphicsView(scene);
view->show();
```
现在你就可以在场景上看到一个矩形了。如果你想对矩形进行更多的自定义,可以使用QPen和QBrush类来设置线条和填充颜色。例如,下面的代码将矩形的边框颜色设置为红色,填充颜色设置为蓝色:
```
QPen pen(Qt::red);
QBrush brush(Qt::blue);
rect->setPen(pen);
rect->setBrush(brush);
```
希望这能帮助你在Qt场景上绘制矩形。
相关问题
qt 怎么用QPainter 在scene上绘制矩形
要在`QGraphicsScene`上绘制矩形,可以使用`QGraphicsScene`提供的`addRect()`方法,也可以使用`QPainter`在`QGraphicsScene`上绘制矩形。
以下是使用`QPainter`在`QGraphicsScene`上绘制矩形的示例代码:
```cpp
QGraphicsScene* scene = new QGraphicsScene();
QGraphicsView* view = new QGraphicsView(scene);
// 在scene上添加一个矩形item
QGraphicsRectItem* rectItem = new QGraphicsRectItem(0, 0, 100, 100);
scene->addItem(rectItem);
// 获取矩形item的边界矩形
QRectF rect = rectItem->boundingRect();
// 创建一个QPainter
QPainter painter;
painter.begin(scene);
// 在scene的(50, 50)位置绘制一个矩形
painter.drawRect(50, 50, rect.width(), rect.height());
painter.end();
```
以上代码中,我们先在`QGraphicsScene`上添加了一个矩形`QGraphicsRectItem`,然后使用`QPainter`在`QGraphicsScene`上绘制了一个矩形,绘制的位置是矩形item的中心点(50, 50)。需要注意的是,我们在绘制前需要调用`QPainter::begin()`方法,绘制完成后需要调用`QPainter::end()`方法。
qt怎么实现鼠标实时在scene上画矩形
要在Qt的scene上实时画矩形,可以采用以下步骤:
1. 创建一个继承自QGraphicsScene的类,重载它的mousePressEvent()、mouseMoveEvent()和mouseReleaseEvent()函数。
2. 在mousePressEvent()函数中,记录鼠标按下的位置,并创建一个QGraphicsRectItem对象。
3. 在mouseMoveEvent()函数中,计算鼠标移动的距离,并更新QGraphicsRectItem的位置和大小。
4. 在mouseReleaseEvent()函数中,将QGraphicsRectItem添加到场景中,并将其置空。
下面是一个简单的示例代码:
```cpp
class MyScene : public QGraphicsScene
{
public:
MyScene(QObject* parent = 0) : QGraphicsScene(parent), rectItem(nullptr) {}
protected:
void mousePressEvent(QGraphicsSceneMouseEvent* event) override
{
startPoint = event->scenePos();
rectItem = new QGraphicsRectItem(startPoint.x(), startPoint.y(), 0, 0);
addItem(rectItem);
}
void mouseMoveEvent(QGraphicsSceneMouseEvent* event) override
{
if (rectItem)
{
qreal x = std::min(startPoint.x(), event->scenePos().x());
qreal y = std::min(startPoint.y(), event->scenePos().y());
qreal w = std::abs(event->scenePos().x() - startPoint.x());
qreal h = std::abs(event->scenePos().y() - startPoint.y());
rectItem->setRect(x, y, w, h);
}
}
void mouseReleaseEvent(QGraphicsSceneMouseEvent* event) override
{
if (rectItem)
{
rectItem = nullptr;
}
}
private:
QPointF startPoint;
QGraphicsRectItem* rectItem;
};
```
在上面的代码中,我们在mousePressEvent()函数中记录了鼠标按下的位置,创建了一个QGraphicsRectItem对象,并将其添加到场景中。在mouseMoveEvent()函数中,我们计算了鼠标移动的距离,并更新了QGraphicsRectItem的位置和大小。在mouseReleaseEvent()函数中,我们将QGraphicsRectItem置空,以便下次绘制。
相关推荐
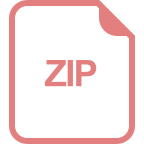
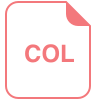
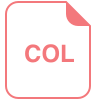
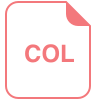









