.定义一个存放在包com.inter下的接口Sort,接口中定义了排序方法sort()。编写该接口的实现类,实现选择排序ChoiceSort和冒泡排序BubbleSort,分别实现对一组整数的降序排列。再编写一个测试类TestSort,在测试类的主方法中通过Sort对象(接口回调)调用方法sort(),分别实现对一组整数的选择算法和冒泡算法的降序排列并输出排序结果。
时间: 2024-02-01 11:15:57 浏览: 57
首先,创建一个Java项目,项目名为SortDemo,包名为com.inter。
在com.inter包下创建一个名为Sort的接口,代码如下:
```
package com.inter;
public interface Sort {
void sort(int[] arr);
}
```
接口中定义了一个sort方法,用于排序。
然后,分别创建ChoiceSort和BubbleSort两个类,实现Sort接口,完成选择排序和冒泡排序的实现。代码如下:
```
package com.inter;
public class ChoiceSort implements Sort {
@Override
public void sort(int[] arr) {
int temp;
for (int i = 0; i < arr.length - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] > arr[minIndex]) {
minIndex = j;
}
}
if (minIndex != i) {
temp = arr[i];
arr[i] = arr[minIndex];
arr[minIndex] = temp;
}
}
}
}
```
```
package com.inter;
public class BubbleSort implements Sort {
@Override
public void sort(int[] arr) {
int temp;
for (int i = 0; i < arr.length - 1; i++) {
for (int j = 0; j < arr.length - 1 - i; j++) {
if (arr[j] < arr[j + 1]) {
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
}
```
在com.inter包下创建一个名为TestSort的测试类,用于测试选择排序和冒泡排序的实现。在主方法中,通过Sort对象(接口回调)调用方法sort(),分别实现对一组整数的选择算法和冒泡算法的降序排列并输出排序结果。代码如下:
```
package com.inter;
import java.util.Arrays;
public class TestSort {
public static void main(String[] args) {
int[] arr = {5, 3, 8, 6, 4};
Sort choiceSort = new ChoiceSort();
choiceSort.sort(arr);
System.out.println("选择排序结果:" + Arrays.toString(arr));
int[] arr2 = {5, 3, 8, 6, 4};
Sort bubbleSort = new BubbleSort();
bubbleSort.sort(arr2);
System.out.println("冒泡排序结果:" + Arrays.toString(arr2));
}
}
```
运行TestSort类,可以看到以下输出:
```
选择排序结果:[8, 6, 5, 4, 3]
冒泡排序结果:[8, 6, 5, 4, 3]
```
注意:选择排序和冒泡排序都是按照降序排列实现的。如果要按照升序排列,只需要修改比较符号即可。
阅读全文
相关推荐
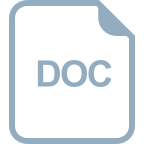
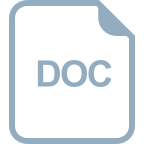
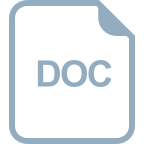
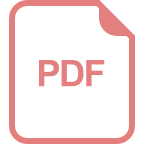
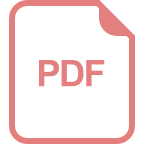
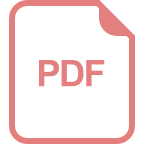
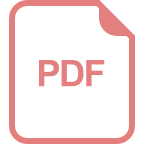
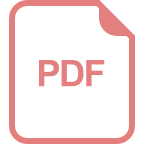
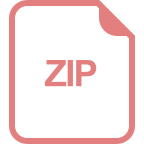
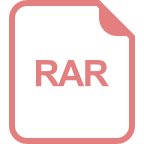
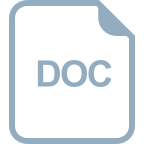
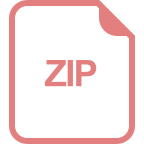
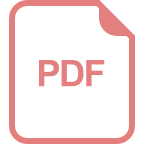
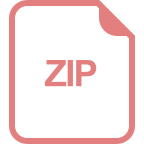
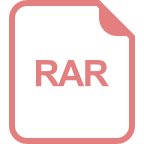
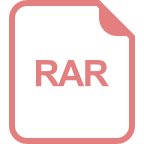
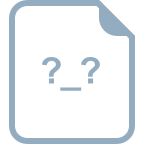
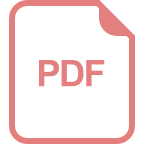
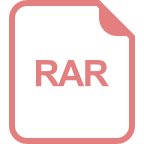