咱们在python中安装ConvexHull库
时间: 2024-04-22 20:24:43 浏览: 103
在Python中,`ConvexHull`函数是`scipy.spatial`模块中的一部分,因此我们需要安装`scipy`库来使用它。
安装`scipy库的方法有多种,最常用方法是使用pip命令。请按照以下步骤进行安装:
1. 打开终端或命令提示符。
2. 运行以下命令来安装`scipy`库:
```
pip install scipy
```
3. 等待安装完成。一旦安装完成,您就可以在Python中使用`ConvexHull`函数了。
请注意,如果您使用的是Anaconda环境,则可以使用以下命令来安装`scipy`库:
```
conda install scipy
```
完成安装后,您就可以在您的代码中导入并使用`ConvexHull`函数了。
相关问题
利用Python编程,使用OpenCV库,获取各工件轮廓并绘制在原图中,识别出工件形状类型:三角形、正方形和平行四边形。
在Python中利用OpenCV库处理工件轮廓并识别形状,首先你需要读取图片,然后进行二值化、边缘检测,接着找出轮廓,最后通过计算轮廓的几何特性来判断形状。以下是一个基本步骤的示例代码:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
def get_shape_type(cnt):
peri = cv2.arcLength(cnt, True)
approx = cv2.approxPolyDP(cnt, 0.04 * peri, True)
if len(approx) == 3:
return "三角形"
elif len(approx) == 4 and cv2.isContourConvex(approx):
# 检查是否为正方形,正方形四个顶点的距离应该相等
sides = [cv2.norm(approx[i] - approx[(i + 1) % 4]) for i in range(4)]
if abs(max(sides) - min(sides)) < 1e-6:
return "正方形"
elif len(approx) == 4 and not cv2.isContourConvex(approx): # 平行四边形
return "平行四边形"
else:
return "未知形状"
# 读取图片
img = cv2.imread('your_image.jpg', 0) # 黑白模式
# 归一化
ret, thresh = cv2.threshold(img, 127, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)
# 寻找轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 绘制轮廓并识别形状
for cnt in contours:
shape_type = get_shape_type(cnt)
cv2.drawContours(img, [cnt], -1, (0, 255, 0), 2) # 绿色边框
cv2.putText(img, shape_type, tuple(cv2.convexHull(cnt)[0][0]), cv2.FONT_HERSHEY_SIMPLEX, 0.8, (0, 255, 0), 2)
plt.imshow(img, cmap='gray')
plt.show()
```
这个例子中,我们首先对图像进行二值化处理,然后寻找轮廓。`get_shape_type`函数会检查轮廓的顶点数量,并基于长度和凸性来确定形状类型。
python中求最小外接矩的顶点
在Python中,可以使用旋转卡壳算法(Rotating Calipers algorithm)来求解最小外接矩形的顶点。
首先,我们需要找到凸包(Convex Hull)的顶点。可以使用Scipy库中的ConvexHull函数来实现这一步骤:
```python
from scipy.spatial import ConvexHull
# 假设points是一个包含点坐标的列表或数组
hull = ConvexHull(points)
hull_vertices = hull.vertices
```
接下来,我们需要计算凸包中每个边向量的方向。可以使用numpy库来进行向量的计算:
```python
import numpy as np
def calculate_direction(point1, point2):
vector = np.array([point2[0] - point1[0], point2[1] - point1[1]])
normalized_vector = vector / np.linalg.norm(vector)
return normalized_vector
directions = []
for i in range(len(hull_vertices)):
point1 = points[hull_vertices[i]]
point2 = points[hull_vertices[(i+1)%len(hull_vertices)]]
direction = calculate_direction(point1, point2)
directions.append(direction)
```
然后,我们需要计算每个边向量所对应的垂直向量,即旋转90度。可以使用numpy库中的dot和cross函数来实现向量的旋转:
```python
def calculate_perpendicular(vector):
perpendicular_vector = np.dot(vector, [[0, -1], [1, 0]])
return perpendicular_vector
perpendiculars = [calculate_perpendicular(direction) for direction in directions]
```
最后,我们需要遍历凸包的每个顶点,并根据其对应的垂直向量找到最小外接矩形的顶点:
```python
min_area = float('inf')
min_rectangle_vertices = []
for i in range(len(hull_vertices)):
vertex = points[hull_vertices[i]]
perpendicular = perpendiculars[i]
min_projection = float('inf')
max_projection = float('-inf')
min_vertex = None
max_vertex = None
for j in range(len(points)):
projection = np.dot(points[j] - vertex, perpendicular)
if projection < min_projection:
min_projection = projection
min_vertex = points[j]
if projection > max_projection:
max_projection = projection
max_vertex = points[j]
area = abs(min_projection) * abs(max_projection)
if area < min_area:
min_area = area
min_rectangle_vertices = [min_vertex, max_vertex]
```
通过以上的代码,变量`min_rectangle_vertices`将会包含最小外接矩形的两个顶点。请注意,这里假设`points`是包含所有点坐标的列表或数组。如果你有其他的数据结构,请相应地修改代码。
希望能帮到你!如果有任何问题,请随时提问。
阅读全文
相关推荐





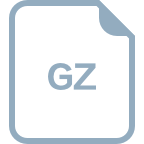
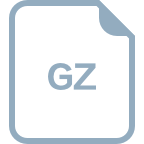







