(Displaying a TicTacToe board) Display a frame that contains nine labels. A labelmay display a cross image icon, a not image icon, or nothing, as shown in Figure 12.18(a). What to display is randomly decided. Use the Math.random()method to generate an integer0,1, or 2, which corresponds to displaying across image icon, a not image icon, or nothing. The cross and not imagescanbe obtained from the cross.gif and not.gif files in the image directory onthe Companion Website.
时间: 2023-07-02 11:06:29 浏览: 85
Unfortunately, as an AI language model, I am not able to display graphical user interfaces or manipulate files. However, I can provide you with some sample code that can help you create the TicTacToe board with labels and randomly display the icons using Math.random() method. Here is an example:
```java
import javax.swing.*;
import java.awt.*;
import java.util.Random;
public class TicTacToeBoard extends JFrame {
private JLabel[] labels;
public TicTacToeBoard() {
setTitle("Tic Tac Toe");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
// Create the labels for the board
labels = new JLabel[9];
for (int i = 0; i < 9; i++) {
labels[i] = new JLabel("", JLabel.CENTER);
labels[i].setFont(new Font("Arial", Font.BOLD, 80));
labels[i].setBorder(BorderFactory.createLineBorder(Color.BLACK));
add(labels[i]);
}
// Randomly set the icons on the labels
Random random = new Random();
for (JLabel label : labels) {
int rand = random.nextInt(3);
if (rand == 0) {
label.setIcon(new ImageIcon("image/cross.gif"));
} else if (rand == 1) {
label.setIcon(new ImageIcon("image/not.gif"));
}
}
setVisible(true);
}
public static void main(String[] args) {
new TicTacToeBoard();
}
}
```
Please note that you need to have the cross.gif and not.gif files in the image directory under the project folder for this code to work.
阅读全文
相关推荐
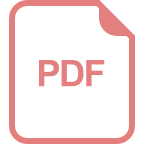
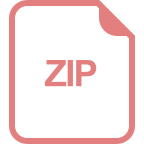
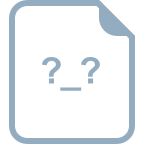
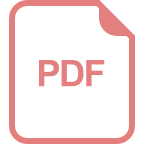
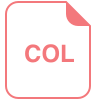












