C#托管代码与非托管代码的交互,DllImport静态入口方法的第一个参数是指定C++的dll路径,必须是常量,这个时候如果我不想 使用绝对路径,那么在C#中如何指定常量的路径
时间: 2024-12-23 12:16:49 浏览: 15
在C#中,与非托管代码(如C++的DLL)交互时,通常使用`DllImport`属性来导入非托管函数。`DllImport`的第一个参数是指定DLL路径的字符串,这个路径必须是常量。因此,如果你不想使用绝对路径,可以通过以下几种方式来指定相对路径或动态生成路径:
1. **使用相对路径**:
如果DLL文件位于应用程序的执行目录或其子目录中,可以使用相对路径。例如,如果DLL文件位于应用程序的根目录下,可以这样写:
```csharp
[DllImport("mylibrary.dll")]
public static extern void MyFunction();
```
2. **使用环境变量**:
可以将DLL文件的路径存储在一个环境变量中,然后在运行时读取该环境变量并构建路径。例如:
```csharp
string dllPath = Environment.GetEnvironmentVariable("MY_LIBRARY_PATH");
if (!string.IsNullOrEmpty(dllPath))
{
[DllImport(dllPath)]
public static extern void MyFunction();
}
```
3. **配置文件**:
将DLL文件的路径存储在配置文件(如App.config或Web.config)中,并在运行时读取该配置项。例如:
```xml
<!-- App.config -->
<configuration>
<appSettings>
<add key="MyLibraryPath" value="relative\path\to\mylibrary.dll"/>
</appSettings>
</configuration>
```
然后在C#代码中读取配置项:
```csharp
string dllPath = ConfigurationManager.AppSettings["MyLibraryPath"];
if (!string.IsNullOrEmpty(dllPath))
{
[DllImport(dllPath)]
public static extern void MyFunction();
}
```
4. **动态加载DLL**:
使用`LoadLibrary`和`GetProcAddress`方法动态加载DLL并获取函数指针。这种方法更灵活,但需要更多的代码。例如:
```csharp
using System;
using System.Runtime.InteropServices;
class Program
{
[DllImport("kernel32.dll", SetLastError = true, CharSet = CharSet.Auto)]
static extern IntPtr LoadLibrary(string lpFileName);
[DllImport("kernel32.dll", CharSet = CharSet.Ansi, ExactSpelling = true, SetLastError = true)]
static extern IntPtr GetProcAddress(IntPtr hModule, string procName);
[DllImport("kernel32.dll", SetLastError = true)]
static extern bool FreeLibrary(IntPtr hModule);
delegate void MyFunctionDelegate();
static void Main()
{
string dllPath = "relative\\path\\to\\mylibrary.dll"; // 或者从配置文件/环境变量中读取路径
IntPtr hModule = LoadLibrary(dllPath);
if (hModule != IntPtr.Zero)
{
IntPtr pFunc = GetProcAddress(hModule, "MyFunction");
if (pFunc != IntPtr.Zero)
{
MyFunctionDelegate myFunction = (MyFunctionDelegate)Marshal.GetDelegateForFunctionPointer(pFunc, typeof(MyFunctionDelegate));
myFunction();
}
FreeLibrary(hModule);
}
}
}
```
通过以上方法,可以在不使用绝对路径的情况下,灵活地指定DLL文件的路径,从而实现C#与非托管代码的交互。
阅读全文
相关推荐




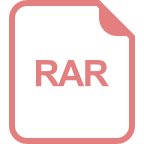
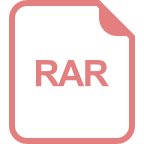




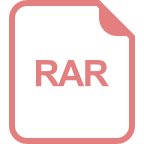





