用c++编写程序,要求首先输入学生人数(最多为50个人),然后按编号从小到大的-|||-依次输入学生成绩(包括语文、数学和英语3门课程的成绩),最后统计每门课程全班-|||-成绩和平均成绩以及每个学生课程的总成绩和平均成绩。
时间: 2024-10-09 17:05:53 浏览: 36
在C++中,你可以使用数组或动态数据结构如vector来存储学生信息,并遍历输入以计算成绩和统计数据。以下是一个简单的示例代码框架:
```cpp
#include <iostream>
#include <vector>
using namespace std;
struct Student {
int id;
int chinese; // 语文成绩
int math; // 数学成绩
int english; // 英语成绩
double totalScore() { return chinese + math + english; }
double averageScore() { return (totalScore()) / 3.0; }
};
int main() {
const int MAX_STUDENTS = 50;
vector<Student> students(MAX_STUDENTS);
int numStudents;
cout << "请输入学生人数(不超过50人):" << endl;
cin >> numStudents;
if (numStudents > MAX_STUDENTS) {
cout << "错误:学生人数超过限制!" << endl;
return 1;
}
for (int i = 0; i < numStudents; ++i) {
cout << "请输入学生" << i+1 << "的编号及各科成绩:" << endl;
cin >> students[i].id;
cin >> students[i].chinese >> students[i].math >> students[i].english;
// 按照编号排序,这里假设编号已经从小到大输入
sort(students.begin(), students.end(), [](const Student& a, const Student& b) {
return a.id < b.id;
});
}
// 统计并打印每门课程的全班成绩和平均成绩
int totalChinese = 0, totalMath = 0, totalEnglish = 0;
for (Student student : students) {
totalChinese += student.chinese;
totalMath += student.math;
totalEnglish += student.english;
cout << "学生" << student.id << "的总成绩: " << student.totalScore()
<< ", 平均成绩: " << student.averageScore() << endl;
}
cout << "全班语文总成绩: " << totalChinese << ", 平均分: "
<< static_cast<double>(totalChinese) / numStudents << endl;
cout << "全班数学总成绩: " << totalMath << ", 平均分: "
<< static_cast<double>(totalMath) / numStudents << endl;
cout << "全班英语总成绩: " << totalEnglish << ", 平均分: "
<< static_cast<double>(totalEnglish) / numStudents << endl;
return 0;
}
```
阅读全文
相关推荐
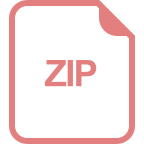
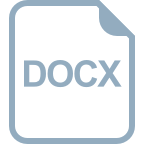
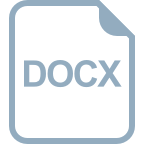


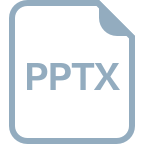


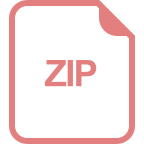
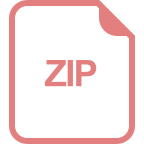
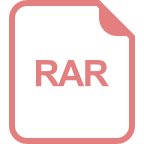
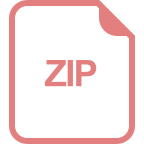
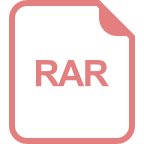
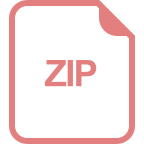
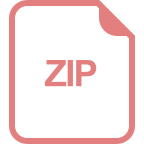