任务:为一家饮品店设计、实现饮品结算功能。该店有coffee、tea两种饮品,coffee可添加suger、milk等辅料,tea可以添加lemon、orange等辅料,辅料同样需要计算价格。Java
时间: 2024-10-16 15:07:02 浏览: 6
为一家饮品店设计并实现饮品结算功能是一个常见的需求。在Java中,可以通过面向对象编程(OOP)和设计模式来实现这一功能。以下是一个简单的示例,说明如何通过Java类和方法来实现这个功能。
首先,定义基础的饮品类 `Drink` 和一些具体的饮品类 `Coffee` 和 `Tea`。然后,使用装饰者模式来处理辅料的添加。
```java
// 基础饮品类
abstract class Drink {
String name;
double price;
public Drink(String name, double price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
}
// Coffee 类
class Coffee extends Drink {
public Coffee() {
super("Coffee", 20.0); // 基础价格
}
}
// Tea 类
class Tea extends Drink {
public Tea() {
super("Tea", 15.0); // 基础价格
}
}
// 辅料类,实现装饰者模式
interface Condiment {
public double getPrice();
public String getDescription();
}
// Sugar 辅料
class Sugar implements Condiment {
public double getPrice() {
return 2.0;
}
public String getDescription() {
return " + Sugar";
}
}
// Milk 辅料
class Milk implements Condiment {
public double getPrice() {
return 3.0;
}
public String getDescription() {
return " + Milk";
}
}
// Lemon 辅料
class Lemon implements Condiment {
public double getPrice() {
return 1.5;
}
public String getDescription() {
return " + Lemon";
}
}
// Orange 辅料
class Orange implements Condiment {
public double getPrice() {
return 2.0;
}
public String getDescription() {
return " + Orange";
}
}
// 装饰器类,用于将辅料添加到饮品中
class DrinkWithCondiment extends Drink {
private Drink drink;
private List<Condiment> condiments;
public DrinkWithCondiment(Drink drink) {
this.drink = drink;
this.condiments = new ArrayList<>();
}
public void addCondiment(Condiment condiment) {
condiments.add(condiment);
}
@Override
public String getName() {
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append(drink.getName());
for (Condiment condiment : condiments) {
stringBuilder.append(condiment.getDescription());
}
return stringBuilder.toString();
}
@Override
public double getPrice() {
double total = drink.getPrice();
for (Condiment condiment : condiments) {
total += condiment.getPrice();
}
return total;
}
}
// 测试代码
public class Main {
public static void main(String[] args) {
Drink coffee = new Coffee();
Drink tea = new Tea();
DrinkWithCondiment coffeePlusSugar = new DrinkWithCondiment(coffee);
coffeePlusSugar.addCondiment(new Sugar());
System.out.println(coffeePlusSugar.getName() + ": " + coffeePlusSugar.getPrice());
DrinkWithCondiment teaPlusMilkAndLemon = new DrinkWithCondiment(tea);
teaPlusMilkAndLemon.addCondiment(new Milk());
teaPlusMilkAndLemon.addCondiment(new Lemon());
System.out.println(teaPlusMilkAndLemon.getName() + ": " + teaPlusMilkAndLemon.getPrice());
}
}
```
在这个示例中:
- `Drink` 是基础饮品类,包含名称和价格。
- `
阅读全文
相关推荐
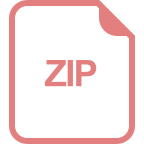
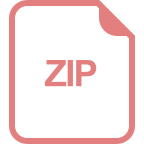
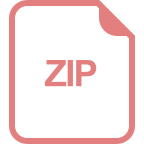

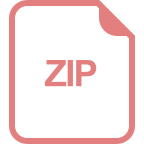
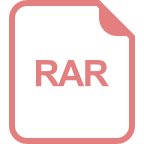
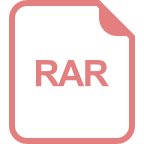
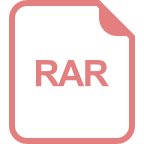
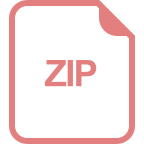









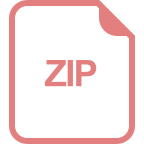