c# 在image上绘制直线
时间: 2024-09-23 20:02:44 浏览: 33
在C#中,特别是在Windows Presentation Foundation (WPF) 或 Universal Windows Platform (UWP) 中,你可以使用System.Drawing命名空间下的Graphics类或者DrawingVisual、DrawingContext等类来在Image控件上绘制直线。以下是使用Graphics类的基本步骤:
```csharp
using System.Windows;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
// 创建一个UserControl
public partial class DrawLineOnImage : UserControl
{
private Image image;
private DrawingVisual drawingVisual;
private RenderTargetBitmap renderBitmap;
public DrawLineOnImage()
{
InitializeComponent();
// 初始化图像控件
image = new Image();
image.Source = new BitmapImage(new Uri("path_to_your_image.jpg")); // 替换为你图片的实际路径
this.Content = image;
// 初始化绘图上下文
drawingVisual = new DrawingVisual();
drawingVisual.RenderTransform = image.RenderTransform;
drawingContext = drawingVisual.RenderOpen();
// 绘制直线
var pen = new Pen(Brushes.Black, 2); // 设置笔的颜色和宽度
drawingContext.DrawLine(pen, new Point(0, 0), new Point(image.Width, image.Height)); // 从左上角画到底部右角
}
protected override void OnRender(DrawingContext drawingContext)
{
base.OnRender(drawingContext);
// 将图像转为位图,以便可以在上面绘图
if (renderBitmap == null || renderBitmap.PixelWidth != image.ActualWidth || renderBitmap.PixelHeight != image.ActualHeight)
{
renderBitmap = new RenderTargetBitmap((int)image.ActualWidth, (int)image.ActualHeight, 96, 96, PixelFormats.Pbgra32);
renderBitmap.Render(drawingVisual);
}
// 渲染到UI
drawingContext.DrawImage(renderBitmap, new Rect(0, 0, image.ActualWidth, image.ActualHeight));
}
}
```
在这个例子中,我们创建了一个用户控件,它包含一个Image控件,用于显示原始图像。当控件渲染时,我们会在画布上绘制一条线,然后再将结果渲染回Image。
阅读全文
相关推荐
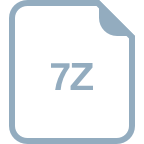
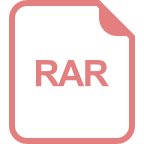
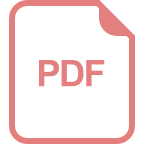















