java语言实现选择、判断题目考试系统,要求程序预设5个选择题及答案,显示在GUI界面中,答题者按照题目内容进行答题,点击按钮提交后可以看到自己的答题结果。 界面要示:图形界面实现。具体代码请给出
时间: 2023-12-14 19:36:08 浏览: 182
以下是一个简单的Java程序实现选择题目考试系统的代码,使用了Swing库实现GUI界面:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ExamSystem extends JFrame implements ActionListener {
private JLabel title, question1, question2, question3, question4, question5;
private JRadioButton q1a, q1b, q1c, q1d, q2a, q2b, q2c, q2d, q3a, q3b, q3c, q3d, q4a, q4b, q4c, q4d, q5a, q5b, q5c, q5d;
private JButton submit;
private ButtonGroup group1, group2, group3, group4, group5;
public ExamSystem() {
// 设置窗口标题和大小
setTitle("选择题考试系统");
setSize(600, 400);
// 创建主题标签
title = new JLabel("选择题考试系统");
title.setFont(new Font("宋体", Font.BOLD, 24));
title.setHorizontalAlignment(JLabel.CENTER);
// 创建题目标签和选项按钮
question1 = new JLabel("1. Java中用于创建对象的关键字是:");
question2 = new JLabel("2. Java中的继承是通过什么关键字实现的?");
question3 = new JLabel("3. 下列哪个不是Java的访问修饰符?");
question4 = new JLabel("4. Java中如何获取当前系统时间?");
question5 = new JLabel("5. Java中的集合框架是用来做什么的?");
q1a = new JRadioButton("new", false);
q1b = new JRadioButton("alloc", false);
q1c = new JRadioButton("create", false);
q1d = new JRadioButton("instanceof", false);
group1 = new ButtonGroup();
group1.add(q1a);
group1.add(q1b);
group1.add(q1c);
group1.add(q1d);
q2a = new JRadioButton("extends", false);
q2b = new JRadioButton("implements", false);
q2c = new JRadioButton("inherits", false);
q2d = new JRadioButton("derives", false);
group2 = new ButtonGroup();
group2.add(q2a);
group2.add(q2b);
group2.add(q2c);
group2.add(q2d);
q3a = new JRadioButton("public", false);
q3b = new JRadioButton("private", false);
q3c = new JRadioButton("protected", false);
q3d = new JRadioButton("friendly", false);
group3 = new ButtonGroup();
group3.add(q3a);
group3.add(q3b);
group3.add(q3c);
group3.add(q3d);
q4a = new JRadioButton("Date.now()", false);
q4b = new JRadioButton("System.currentTimeMillis()", false);
q4c = new JRadioButton("Clock.now()", false);
q4d = new JRadioButton("Time.now()", false);
group4 = new ButtonGroup();
group4.add(q4a);
group4.add(q4b);
group4.add(q4c);
group4.add(q4d);
q5a = new JRadioButton("存储数据", false);
q5b = new JRadioButton("读取数据", false);
q5c = new JRadioButton("排序数据", false);
q5d = new JRadioButton("操作数据", false);
group5 = new ButtonGroup();
group5.add(q5a);
group5.add(q5b);
group5.add(q5c);
group5.add(q5d);
// 创建提交按钮
submit = new JButton("提交");
submit.addActionListener(this);
// 添加组件到面板中
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(12, 1));
panel.add(title);
panel.add(question1);
panel.add(q1a);
panel.add(q1b);
panel.add(q1c);
panel.add(q1d);
panel.add(question2);
panel.add(q2a);
panel.add(q2b);
panel.add(q2c);
panel.add(q2d);
panel.add(question3);
panel.add(q3a);
panel.add(q3b);
panel.add(q3c);
panel.add(q3d);
panel.add(question4);
panel.add(q4a);
panel.add(q4b);
panel.add(q4c);
panel.add(q4d);
panel.add(question5);
panel.add(q5a);
panel.add(q5b);
panel.add(q5c);
panel.add(q5d);
panel.add(submit);
// 将面板添加到窗口中
add(panel);
// 设置窗口可见
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
// 计算得分
int score = 0;
if (q1c.isSelected()) score++;
if (q2a.isSelected()) score++;
if (q3d.isSelected()) score++;
if (q4b.isSelected()) score++;
if (q5d.isSelected()) score++;
// 显示得分
JOptionPane.showMessageDialog(null, "你的得分是:" + score + "/5");
}
public static void main(String[] args) {
new ExamSystem();
}
}
```
该程序将题目、选项和提交按钮添加到面板中,并在提交按钮被点击时计算得分并显示结果。
阅读全文
相关推荐


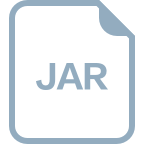


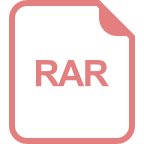
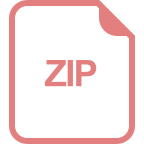


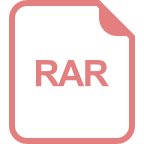








