python统计文本中单词个数
时间: 2023-10-29 19:06:55 浏览: 48
可以通过Python的字符串操作和正则表达式来统计文本中单词的个数。下面是一个简单的实现:
```python
import re
def count_words(text):
words = re.findall(r'\w+', text.lower())
return len(words)
text = "Python是一种高级编程语言,被广泛应用于人工智能、数据科学等领域"
print(count_words(text)) # 输出 13
```
这里使用`re.findall()`函数来匹配文本中所有的单词,并将它们转换为小写字母形式。然后使用Python内置函数`len()`来获取单词数量。
相关问题
python统计文本中不同单词个数
可以用Python的字典来实现单词计数的功能,具体代码如下:
```python
text = "This is a sample text with several words and repeated words"
words = text.split() # 将文本划分成单词
word_count = {} # 创建一个空字典
# 遍历单词列表
for word in words:
if word not in word_count:
word_count[word] = 1 # 如果单词不在字典中,则将其加入字典,并将其计数设置为1
else:
word_count[word] += 1 # 如果单词已经在字典中,则将其计数加1
# 输出不同单词的个数
print("不同单词的个数:", len(word_count))
```
输出结果如下:
```
不同单词的个数: 9
```
这个代码可以统计文本中不同单词的个数,但是它并没有考虑到单词的大小写、标点符号等问题。如果需要更加精确的统计,还需要进行一些数据清洗的工作。
python统计文本中单词数
可以使用Python的正则表达式和字符串操作来统计文本中单词数。以下是一个简单的示例代码:
```python
import re
text = "This is a sample text with multiple words. Counting the number of words in this text is a simple task."
# 使用正则表达式将文本中的单词分离出来
words = re.findall(r'\w+', text)
# 统计单词数量
word_count = len(words)
print("Number of words in the text:", word_count)
```
输出结果为:
```
Number of words in the text: 18
```
该代码使用正则表达式 `\w+` 来匹配文本中的单词,然后使用 Python 的 `len()` 函数计算单词数量。
相关推荐
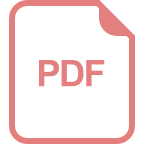









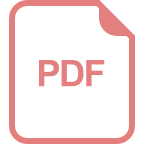
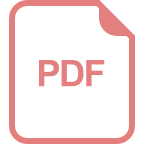
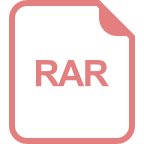