统计文本中的单词数(Python)
时间: 2024-06-24 17:00:45 浏览: 29
在Python中,统计文本中的单词数通常涉及到将文本分解为单词并计算它们的数量。你可以使用内置的字符串方法和正则表达式库`re`来实现这个功能。以下是一个简单的示例:
```python
import re
def count_words(text):
# 使用正则表达式匹配单词,并替换非字母字符为空格
words = re.findall(r'\b\w+\b', text.lower())
# 返回单词数量(不包括标点符号)
return len(words)
# 示例文本
text = "This is a sample sentence, with several words. It contains punctuation marks like commas and periods."
word_count = count_words(text)
print(f"Text has {word_count} words.")
相关推荐
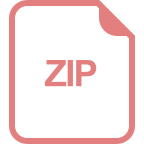
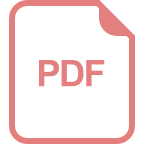
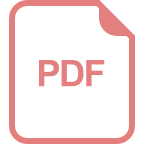
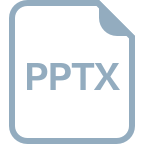