修改程序gray = cv2.imread('gray_high_3.bmp', cv2.IMREAD_GRAYSCALE) matrix = np.array(gray) matrix = matrix / 255,将通过matrix[i, j] = float(values[2]) + 32.45 gray = (matrix * 255).astype(np.uint8)生成的matrix[i,j]恢复为原来的数值
时间: 2024-03-25 07:40:36 浏览: 12
您可以通过如下代码将经过上述操作的matrix恢复为原来的数值:
```python
restored_matrix = (matrix * 255 - 32.45) * (1/255)
```
这里做了两步操作:
1. 首先将matrix乘以255,将其还原为原始的灰度值范围(0-255)。
2. 然后减去32.45,将原来加上的常数去掉。
3. 最后将结果除以255,将其还原为原始的灰度值范围(0-1)。
这样,restored_matrix就是经过恢复后的原始矩阵了。
相关推荐
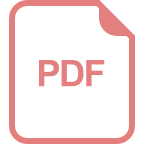
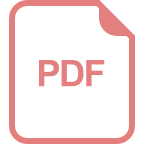
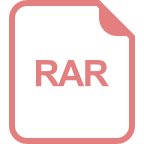
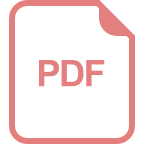
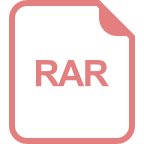
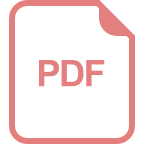
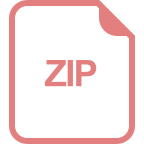
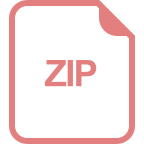
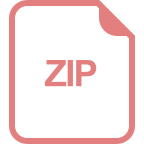
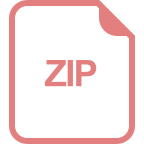
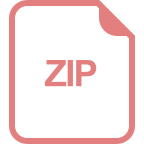
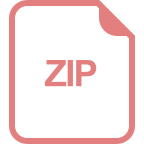
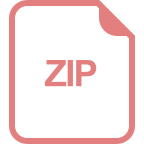
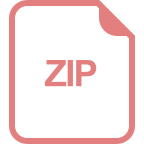
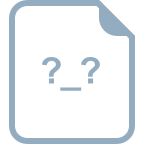