分支界限法 旅行商问题C语言
时间: 2023-08-28 18:07:58 浏览: 69
分支界限法是一种求解问题的通用算法,其中包括解决旅行商问题的算法。以下是一个使用 C 语言实现的分支界限法求解旅行商问题的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define N 4
int graph[N][N] = {
{0, 10, 15, 20},
{10, 0, 35, 25},
{15, 35, 0, 30},
{20, 25, 30, 0}
};
typedef struct {
int path[N];
int level;
int bound;
} Node;
int min(int a, int b) {
return a < b ? a : b;
}
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
int calculateBound(Node node) {
int sum = 0;
for (int i = 0; i < N - 1; i++) {
sum += graph[node.path[i]][node.path[i + 1]];
}
sum += graph[node.path[N - 1]][node.path[0]];
for (int i = 0; i < N; i++) {
if (node.path[i] == -1) {
int min1 = 999, min2 = 999;
for (int j = 0; j < N; j++) {
if (i != j && node.path[j] == -1) {
if (graph[i][j] < min1) {
min2 = min1;
min1 = graph[i][j];
} else if (graph[i][j] < min2) {
min2 = graph[i][j];
}
}
}
sum += min1 + min2;
}
}
return sum;
}
void copyPath(int *dest, int *src) {
for (int i = 0; i < N; i++) {
dest[i] = src[i];
}
}
void printPath(int *path) {
for (int i = 0; i < N; i++) {
printf("%d ", path[i]);
}
printf("\n");
}
void solve() {
Node queue[100];
int head = 0, tail = 0;
int bestPath[N], bestCost = 999;
queue[tail].level = 0;
queue[tail].path[0] = 0;
queue[tail].bound = calculateBound(queue[tail]);
tail++;
while (head != tail) {
Node node = queue[head];
head++;
if (node.level == N - 1) {
int cost = calculateBound(node);
if (cost < bestCost) {
bestCost = cost;
copyPath(bestPath, node.path);
}
} else {
for (int i = node.level + 1; i < N; i++) {
Node childNode = node;
swap(&childNode.path[node.level + 1], &childNode.path[i]);
childNode.level++;
childNode.bound = calculateBound(childNode);
if (childNode.bound < bestCost) {
queue[tail] = childNode;
tail++;
}
}
}
}
printf("Best path: ");
printPath(bestPath);
printf("Best cost: %d\n", bestCost);
}
int main() {
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
if (graph[i][j] == 0) {
graph[i][j] = 999;
}
}
}
int path[N];
for (int i = 0; i < N; i++) {
path[i] = -1;
}
path[0] = 0;
solve();
return 0;
}
```
在这个示例代码中,我们定义了一个 `Node` 结构体来表示分支界限法中的节点,其中包括路径、级别和界限。我们还定义了一些辅助函数来计算路径的界限、复制路径、打印路径等。最后我们在 `main` 函数中初始化路径,然后调用 `solve` 函数来求解旅行商问题。
相关推荐
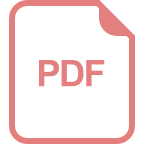



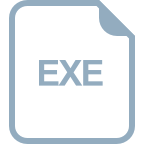
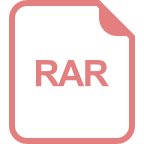
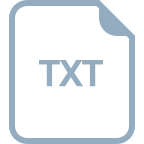
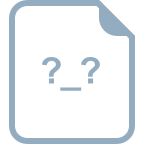