java mysql增删改查
时间: 2023-10-13 09:23:11 浏览: 35
以下是Java中使用MySQL进行增删改查的示例代码:
### 增加数据
```java
public void addStudent(Student student) {
try {
Connection conn = getConnection();
String sql = "INSERT INTO student(name, age, gender) VALUES(?, ?, ?)";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, student.getName());
pstmt.setInt(2, student.getAge());
pstmt.setString(3, student.getGender());
pstmt.executeUpdate();
pstmt.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
### 删除数据
```java
public void deleteStudent(int id) {
try {
Connection conn = getConnection();
String sql = "DELETE FROM student WHERE id=?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
pstmt.executeUpdate();
pstmt.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
### 修改数据
```java
public void updateStudent(Student student) {
try {
Connection conn = getConnection();
String sql = "UPDATE student SET name=?, age=?, gender=? WHERE id=?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, student.getName());
pstmt.setInt(2, student.getAge());
pstmt.setString(3, student.getGender());
pstmt.setInt(4, student.getId());
pstmt.executeUpdate();
pstmt.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
### 查询数据
```java
public List<Student> findAllStudents() {
List<Student> students = new ArrayList<Student>();
try {
Connection conn = getConnection();
String sql = "SELECT * FROM student";
PreparedStatement pstmt = conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
Student student = new Student();
student.setId(rs.getInt("id"));
student.setName(rs.getString("name"));
student.setAge(rs.getInt("age"));
student.setGender(rs.getString("gender"));
students.add(student);
}
rs.close();
pstmt.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
return students;
}
```
以上是Java中使用MySQL进行增删改查的示例代码,其中Student为一个Java Bean类,包含id、name、age、gender等属性。具体实现需要根据实际情况进行调整。
相关推荐
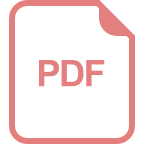














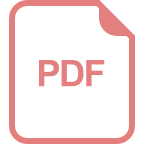