vue3 ts封装axios请求接口
时间: 2023-07-02 22:15:35 浏览: 185
好的,以下是一个简单的 Vue3 + TypeScript 封装 Axios 请求的示例代码:
首先,安装 `axios` 和 `@types/axios`:
```
npm install axios @types/axios --save
```
然后,在 `src/api` 目录下创建 `index.ts` 文件,定义一个 `API` 类来封装 Axios 请求:
```typescript
import axios, { AxiosInstance, AxiosRequestConfig, AxiosResponse } from 'axios';
class API {
private instance: AxiosInstance;
constructor() {
this.instance = axios.create({
baseURL: 'https://your.api.url', // API base URL
timeout: 10000, // Request timeout
});
// Add request interceptor
this.instance.interceptors.request.use(
(config: AxiosRequestConfig) => {
// Do something before request is sent
// Add token to request headers, etc.
return config;
},
(error: any) => {
// Do something with request error
return Promise.reject(error);
},
);
// Add response interceptor
this.instance.interceptors.response.use(
(response: AxiosResponse) => {
// Do something with response data
// Handle error response, etc.
return response.data;
},
(error: any) => {
// Do something with response error
return Promise.reject(error);
},
);
}
public async get<T>(url: string, params?: any, config?: AxiosRequestConfig): Promise<T> {
const response = await this.instance.get<T>(url, { params, ...config });
return response;
}
public async post<T>(url: string, data?: any, config?: AxiosRequestConfig): Promise<T> {
const response = await this.instance.post<T>(url, data, config);
return response;
}
// Add other HTTP methods if needed
}
export const api = new API();
```
在组件中使用 `api` 类,调用 `get` 或 `post` 方法来发送请求:
```typescript
import { defineComponent, ref } from 'vue';
import { api } from '@/api';
export default defineComponent({
setup() {
const data = ref<any>(null);
const fetchData = async () => {
try {
const response = await api.get('/user', { id: 1 });
data.value = response;
} catch (error) {
console.error(error);
}
};
return {
data,
fetchData,
};
},
});
```
以上示例代码中,我们封装了一个 `API` 类来管理 Axios 实例和请求拦截器、响应拦截器等。在组件中,我们通过 `api` 对象来调用 `get` 或 `post` 方法来发送请求,并使用 `async/await` 异步处理响应。
阅读全文
相关推荐
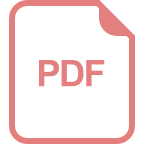
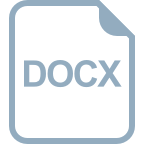
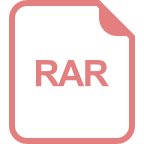















