vue3+ts封装axios全局使用
时间: 2023-08-29 15:13:24 浏览: 62
在Vue3中使用TypeScript和Axios,你可以按照以下步骤完成全局封装:
1. 安装 Axios 和 @types/axios:
```bash
npm install axios @types/axios --save
```
2. 创建 `api.ts` 文件来封装 Axios 请求:
```typescript
import axios, { AxiosInstance, AxiosRequestConfig, AxiosResponse } from 'axios';
interface HttpConfig extends AxiosRequestConfig {
// 自定义配置
}
class Http {
private axiosInstance: AxiosInstance;
constructor() {
this.axiosInstance = axios.create({
// 基础请求路径
baseURL: process.env.BASE_API,
// 请求超时时间
timeout: 5000,
});
// 请求拦截器
this.axiosInstance.interceptors.request.use(
(config: HttpConfig) => {
// 在请求前做些什么
return config;
},
(error: any) => {
// 处理请求错误
Promise.reject(error);
}
);
// 响应拦截器
this.axiosInstance.interceptors.response.use(
(response: AxiosResponse) => {
// 对响应数据做些什么
return response.data;
},
(error: any) => {
// 处理响应错误
Promise.reject(error);
}
);
}
// 封装请求方法
public request<T>(config: HttpConfig): Promise<T> {
return this.axiosInstance.request<T>(config);
}
// 封装 get 请求
public get<T>(url: string, config?: HttpConfig): Promise<T> {
return this.axiosInstance.get<T>(url, config);
}
// 封装 post 请求
public post<T>(url: string, data?: any, config?: HttpConfig): Promise<T> {
return this.axiosInstance.post<T>(url, data, config);
}
// 封装 put 请求
public put<T>(url: string, data?: any, config?: HttpConfig): Promise<T> {
return this.axiosInstance.put<T>(url, data, config);
}
// 封装 delete 请求
public delete<T>(url: string, config?: HttpConfig): Promise<T> {
return this.axiosInstance.delete<T>(url, config);
}
}
export default new Http();
```
3. 在 `main.ts` 中引入 `api.ts` 并挂载到 Vue 实例上:
```typescript
import { createApp } from 'vue';
import App from './App.vue';
import api from './api';
const app = createApp(App);
app.config.globalProperties.$api = api;
app.mount('#app');
```
现在,你就可以在任何组件中使用 `$api` 来调用 Axios 请求了:
```typescript
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
mounted() {
this.$api.get('/api/user').then((res) => {
console.log(res);
});
},
});
</script>
```
相关推荐
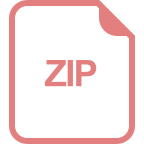
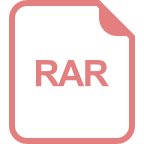
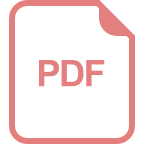
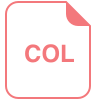
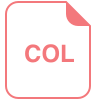
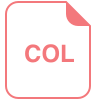
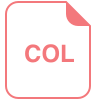
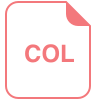









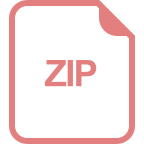