用C语言写一个函数计算链表有多少节点
时间: 2024-03-09 07:46:44 浏览: 16
假设链表的每个节点定义如下:
```
typedef struct Node {
int data; // 节点的数据
struct Node *next; // 指向下一个节点的指针
} Node;
```
则可以写一个计算链表节点个数的函数如下:
```
int countNodes(Node *head) {
int count = 0; // 计数器初始化为0
Node *p = head; // 从链表头节点开始遍历
while (p != NULL) {
count++; // 遍历每个节点时计数器加1
p = p->next; // 指针移动到下一个节点
}
return count; // 返回计数器的值,即链表节点个数
}
```
该函数接受一个指向链表头节点的指针作为参数,返回链表的节点个数。它使用一个计数器变量count,从头节点开始遍历链表,每遇到一个节点就将计数器加1,直到遍历到链表末尾为止。最后返回计数器的值即可。
相关问题
用c语言写一个函数实现把链表写到文件中
可以使用以下代码实现将链表写入文件中:
```c
#include <stdio.h>
struct Node {
int data;
struct Node* next;
};
void writeListToFile(struct Node* head, char* fileName) {
FILE* fp = fopen(fileName, "w");
if (fp == NULL) {
printf("Failed to open file.\n");
return;
}
struct Node* current = head;
while (current != NULL) {
fprintf(fp, "%d ", current->data);
current = current->next;
}
fclose(fp);
}
```
这个函数接受两个参数,一个是链表的头指针,另一个是文件名。它会打开一个文件,将链表中的每个节点的数据写入文件中,每个数据之间用空格分隔。最后,它会关闭文件。
c语言写一个函数insert,用来向一个动态链表插入节点
下面是一个示例代码,实现在动态链表中插入节点的功能:
```c
struct Node {
int data;
struct Node* next;
};
void insert(struct Node** head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
```
在该函数中,我们首先声明一个新的节点 `new_node`,并分配内存空间。然后将新节点的 `data` 值设置为传入的 `new_data` 参数,并将 `next` 指针指向当前链表的头节点 `(*head_ref)`。最后将新节点赋值给 `head_ref`,即可将其插入到链表中。
使用示例:
```c
int main() {
struct Node* head = NULL;
insert(&head, 1);
insert(&head, 2);
insert(&head, 3);
insert(&head, 4);
insert(&head, 5);
return 0;
}
```
上述代码将创建一个包含 5 个节点的动态链表,其中数据值分别为 5、4、3、2、1。
相关推荐
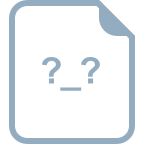
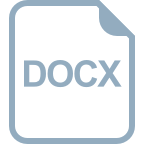
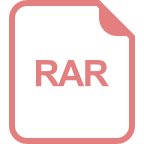












