用c语言写一个循环双链表插入算法
时间: 2023-03-25 13:00:53 浏览: 114
循环双链表插入算法的C语言实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义双向链表节点结构体
typedef struct Node {
int data;
struct Node* prev;
struct Node* next;
} Node;
// 定义循环双向链表结构体
typedef struct List {
Node* head;
Node* tail;
} List;
// 初始化循环双向链表
void initList(List* list) {
list->head = NULL;
list->tail = NULL;
}
// 创建新节点
Node* createNode(int data) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->prev = NULL;
node->next = NULL;
return node;
}
// 在链表尾部插入节点
void insertAtTail(List* list, int data) {
Node* node = createNode(data);
if (list->head == NULL) {
list->head = node;
list->tail = node;
node->prev = node;
node->next = node;
} else {
node->prev = list->tail;
node->next = list->head;
list->tail->next = node;
list->head->prev = node;
list->tail = node;
}
}
// 在链表中间插入节点
void insertAtMiddle(List* list, int data, int position) {
Node* node = createNode(data);
Node* current = list->head;
int i = 1;
while (i < position && current != NULL) {
current = current->next;
i++;
}
if (current == NULL) {
printf("Invalid position\n");
return;
}
node->prev = current->prev;
node->next = current;
current->prev->next = node;
current->prev = node;
}
// 打印链表
void printList(List* list) {
Node* current = list->head;
if (current == NULL) {
printf("List is empty\n");
return;
}
do {
printf("%d ", current->data);
current = current->next;
} while (current != list->head);
printf("\n");
}
int main() {
List list;
initList(&list);
insertAtTail(&list, 1);
insertAtTail(&list, 2);
insertAtTail(&list, 3);
insertAtTail(&list, 4);
insertAtMiddle(&list, 5, 3);
printList(&list);
return ;
}
```
该算法实现了循环双向链表的初始化、在链表尾部插入节点、在链表中间插入节点和打印链表等功能。其中,insertAtMiddle函数实现了在链表中间插入节点的功能,需要传入链表、要插入的数据和插入的位置三个参数。如果插入位置不合法,会输出"Invalid position"。最后,通过调用printList函数打印链表。
阅读全文
相关推荐
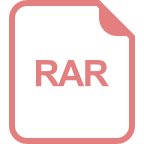
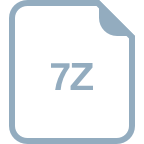
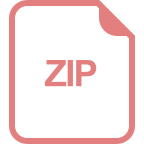















