java aes加密调用
时间: 2023-09-25 11:09:17 浏览: 98
以下是一个 Java 中使用 AES 加密的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class AesEncryption {
private static final String ALGORITHM = "AES";
private static final String KEY = "mysecretkey12345"; // 密钥必须是16个字符长
public static String encrypt(String valueToEncrypt) {
try {
SecretKeySpec secretKeySpec = new SecretKeySpec(KEY.getBytes(StandardCharsets.UTF_8), ALGORITHM);
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec);
byte[] encrypted = cipher.doFinal(valueToEncrypt.getBytes());
return Base64.getEncoder().encodeToString(encrypted);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public static String decrypt(String encryptedValue) {
try {
SecretKeySpec secretKeySpec = new SecretKeySpec(KEY.getBytes(StandardCharsets.UTF_8), ALGORITHM);
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec);
byte[] original = cipher.doFinal(Base64.getDecoder().decode(encryptedValue));
return new String(original);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public static void main(String[] args) {
String valueToEncrypt = "Hello, world!";
String encryptedValue = encrypt(valueToEncrypt);
System.out.println("Encrypted value: " + encryptedValue);
String decryptedValue = decrypt(encryptedValue);
System.out.println("Decrypted value: " + decryptedValue);
}
}
```
这个示例代码中使用了 Base64 编码来将加密后的字节数组转化为字符串,你可以根据实际需求自行修改。
阅读全文
相关推荐
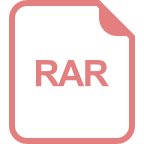
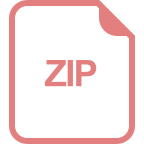
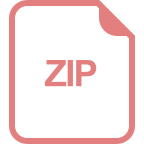
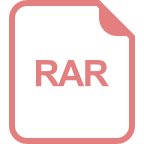
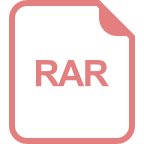
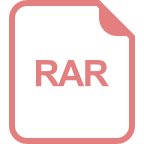
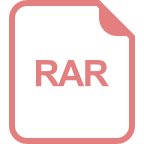
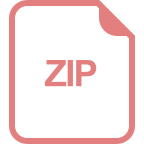
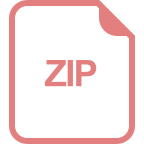
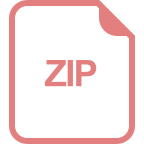
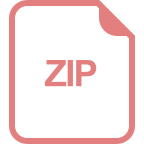
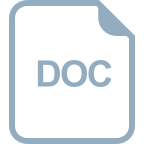
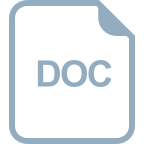
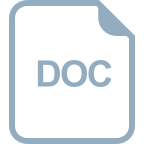
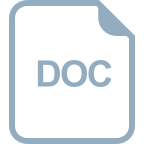
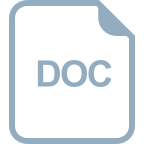