考虑天气,节假日,不考虑三车道,已知天气数据,节假日数据和通过ETC的平均速度,车流量,怎么利用线性插值法计算TPI,给出python代码
时间: 2024-11-15 14:33:45 浏览: 13
为了计算交通压力指数(Traffic Pressure Index, TPI),我们需要结合天气数据、节假日数据以及车流量等因素。这里我们可以使用线性插值法来估算在特定时间段内的平均速度。由于线性插值适用于连续的数据,我们将假定车流量和速度之间存在线性关系。以下是使用Python进行简单线性插值的一个示例:
首先,假设我们有三个变量:`weather_data`, `holiday_data`, 和 `speed_data`,它们对应着天气情况、节假日状态和车速。我们还需要知道给定时间点的`current_weather`, `current_holiday`。
```python
# 假设数据是以列表形式存储的,例如:
weather_data = ... # 天气数据
holiday_data = ... # 节假日数据
speed_data = ... # 平均速度数据
def linear_interpolation(weather, holiday, speed_values, flow_values):
"""
使用线性插值计算给定天气和节日条件下的TPI
"""
# 找到与当前天气和假日最接近的速度和流量值索引
weather_index = bisect.bisect_left(weather_data, current_weather)
holiday_index = bisect.bisect_left(holiday_data, current_holiday)
# 如果索引超出范围,取边界值
if weather_index == len(weather_data):
weather_index -= 1
if holiday_index == len(holiday_data):
holiday_index -= 1
# 计算权重,对于天气和节假日的变化
weight_weather = (current_weather - weather_data[weather_index]) / (weather_data[weather_index + 1] - weather_data[weather_index])
weight_holiday = (current_holiday - holiday_data[holiday_index]) / (holiday_data[holiday_index + 1] - holiday_data[holiday_index])
# 线性插值计算速度
interpolated_speed = speed_values[weather_index] * (1 - weight_weather) + speed_values[weather_index + 1] * weight_weather
# 使用速度和车流量计算TPI,这里假设TPI直接等于速度乘以流量
tpi = interpolated_speed * flow_values[weather_index]
return tpi
# 示例调用
current_weather = ... # 当前天气
current_holiday = ... # 当前节假日
current_flow = ... # 给定时间点的车流量
estimated_tpi = linear_interpolation(current_weather, current_holiday, speed_data, flow_data)
```
请注意,这只是一个基本的实现,实际应用可能需要更复杂的模型来考虑更多的因素,比如非线性关系或缺失数据的处理。另外,`bisect_left`函数用于找到插入位置,它假设输入数据已经排序。
阅读全文
相关推荐





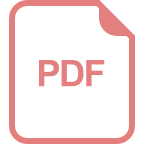







