编写一个函数char * str_bin(char* str1, char* str2), str1、str2是两个有序字符串(其中字符按ASCII码从小到大排序),将str2合并到字符串str1中,要求合并后的字符串仍是有序的,允许字符重复。在main函数中测试该函数:从键盘输入两个有序字符串,然后调用该函数,最后输出合并后的结果。C语言
时间: 2023-06-07 15:07:39 浏览: 110
的代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char * str_bin(char* str1, char* str2);
int main() {
char str1[100], str2[100], *result;
printf("请输入第一个有序字符串:\n");
scanf("%s", str1);
printf("请输入第二个有序字符串:\n");
scanf("%s", str2);
result = str_bin(str1, str2);
printf("合并后的有序字符串为:%s\n", result);
return 0;
}
char * str_bin(char* str1, char* str2) {
int len1 = strlen(str1);
int len2 = strlen(str2);
int i = 0, j = 0, k = 0;
char *result = (char*) malloc(sizeof(char) * (len1 + len2 + 1));
while (i < len1 && j < len2) {
if (str1[i] < str2[j]) {
result[k++] = str1[i++];
} else {
result[k++] = str2[j++];
}
}
while (i < len1) {
result[k++] = str1[i++];
}
while (j < len2) {
result[k++] = str2[j++];
}
result[k] = '\0';
return result;
}
```
上述代码使用了归并排序的思想,先将两个字符串按顺序合并到一个新的字符串中。在合并时,比较两个字符串的当前字符,较小的加入新的字符串中,然后指针往后移动。如果有一个字符串的字符被遍历完了,就将另外一个字符串中剩余的字符加入到新的字符串中。最后返回合并后的字符串即可。
请注意这里的函数返回了一个动态分配的字符数组,需要在使用完毕后手动释放内存,否则会造成内存泄露。
阅读全文
相关推荐
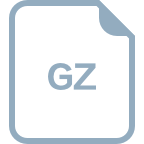
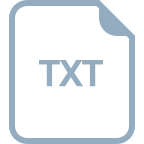
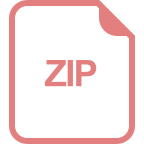
















