对输入的数进行全排列的求解,调用STL模版实现
时间: 2024-03-22 21:41:23 浏览: 63
以下是使用STL模板库实现求解全排列的代码(C++):
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
vector<vector<int>> permute(vector<int>& nums) {
vector<vector<int>> res;
sort(nums.begin(), nums.end()); // 按照字典序排序
do {
res.push_back(nums);
} while (next_permutation(nums.begin(), nums.end())); // 使用STL库中的next_permutation函数生成下一个排列
return res;
}
int main() {
vector<int> nums = {1, 2, 3};
vector<vector<int>> res = permute(nums);
for (auto& row : res) {
for (auto& num : row) {
cout << num << " ";
}
cout << endl;
}
return 0;
}
```
在`permute`函数中,我们首先将输入数组按照字典序排序,然后使用`do-while`循环不断调用`next_permutation`函数生成下一个排列,并将排列加入结果列表中,直到无法生成下一个排列为止。最后返回结果列表即可。
在`main`函数中,我们测试了一下对于输入数组`{1, 2, 3}`的全排列结果。输出如下:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
注意,使用STL模板库需要包含相应的头文件。在本例中,`vector`和`algorithm`头文件均被包含。
阅读全文
相关推荐

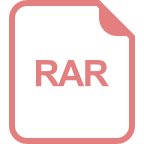
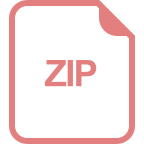
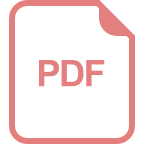
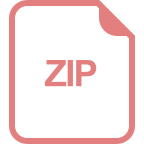
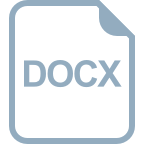
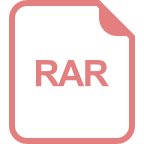
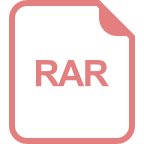
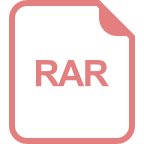
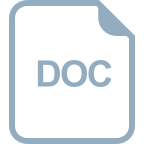
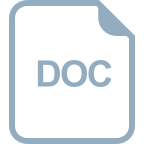
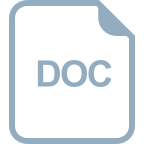
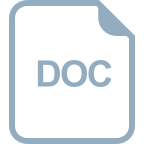
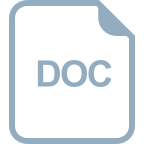
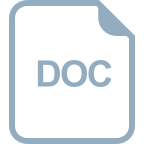
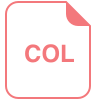