python用EasyEnsemble后样本比例会发生变化吗
时间: 2023-05-31 16:02:20 浏览: 81
是的,使用EasyEnsemble会导致样本比例发生变化。EasyEnsemble是一种基于集成学习的方法,它通过对原始数据集进行随机欠采样来生成多个子集,每个子集都包含原始数据集中的一部分样本。由于欠采样的原因,每个子集中的样本数量都会减少,从而导致样本比例发生变化。通常情况下,EasyEnsemble会生成多个子集,每个子集都包含相同数量的少数类样本和同样数量的随机选择的多数类样本,以实现平衡的样本比例。
相关问题
写一段python代码用EasyEnsemble的方法解决样本欠采样
好的,以下是一个使用EasyEnsemble算法进行样本欠采样的Python代码示例:
```python
from imblearn.ensemble import EasyEnsemble
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
# 创建一个不平衡的二分类数据集
X, y = make_classification(n_samples=10000, n_classes=2, weights=[0.9, 0.1], random_state=42)
# 将数据集分成训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 使用EasyEnsemble算法进行欠采样
ee = EasyEnsemble(n_estimators=10, random_state=42)
X_resampled, y_resampled = ee.fit_resample(X_train, y_train)
# 训练决策树分类器
clf = DecisionTreeClassifier(random_state=42)
clf.fit(X_resampled, y_resampled)
# 在测试集上进行预测
y_pred = clf.predict(X_test)
# 输出预测结果
print(y_pred)
```
在这个示例中,我们使用了`imblearn.ensemble`库中的`EasyEnsemble`算法来进行欠采样。我们首先创建了一个不平衡的二分类数据集,并将其分成训练集和测试集。然后,我们使用EasyEnsemble算法对训练集进行欠采样,生成了多个子数据集。接着,我们使用这些子数据集来训练决策树分类器,并在测试集上进行了预测。最后,我们输出了预测结果。
python用easyensemble和bagging做预测
EasyEnsemble和Bagging是两种集成学习方法,可以用于构建强大的分类器或回归器,其中EasyEnsemble是一种基于集成的方法,而Bagging则是一种基于样本的方法。
下面是使用Python进行EasyEnsemble和Bagging预测的示例代码:
## EasyEnsemble预测
```
from imblearn.ensemble import EasyEnsemble
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.metrics import confusion_matrix
# 生成样本数据
X, y = make_classification(n_samples=1000, n_features=10, n_classes=2, weights=[0.9, 0.1])
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 构建EasyEnsemble分类器
ee = EasyEnsemble(random_state=42)
# 训练模型
ee.fit(X_train, y_train)
# 预测测试集
y_pred = ee.predict(X_test)
# 计算混淆矩阵
cm = confusion_matrix(y_test, y_pred)
print('Confusion Matrix:\n', cm)
```
## Bagging预测
```
from sklearn.ensemble import BaggingClassifier
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.metrics import confusion_matrix
# 生成样本数据
X, y = make_classification(n_samples=1000, n_features=10, n_classes=2, weights=[0.9, 0.1])
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 构建Bagging分类器
bc = BaggingClassifier(base_estimator=DecisionTreeClassifier(), n_estimators=10, random_state=42)
# 训练模型
bc.fit(X_train, y_train)
# 预测测试集
y_pred = bc.predict(X_test)
# 计算混淆矩阵
cm = confusion_matrix(y_test, y_pred)
print('Confusion Matrix:\n', cm)
```
以上代码示例展示了如何使用EasyEnsemble和Bagging进行分类预测,代码中使用了sklearn和imblearn库。在实际应用中,您需要根据自己的数据和需求来选择合适的算法和参数。
相关推荐
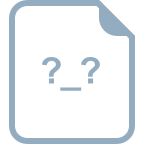
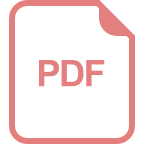
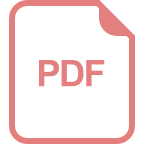












