easyensemble算法的实现python代码
时间: 2024-02-07 22:02:32 浏览: 36
以下是 EasyEnsemble 算法的一个简单实现的 Python 代码示例:
```python
from sklearn.tree import DecisionTreeClassifier
from sklearn.base import clone
from sklearn.utils import resample
import numpy as np
class EasyEnsemble:
def __init__(self, base_classifier=DecisionTreeClassifier(), n_estimators=10):
self.base_classifier = base_classifier
self.n_estimators = n_estimators
self.ensemble = []
def fit(self, X, y):
for _ in range(self.n_estimators):
# 通过自助采样创建一个子集
X_subset, y_subset = resample(X, y)
# 训练基分类器
classifier = clone(self.base_classifier)
classifier.fit(X_subset, y_subset)
# 将基分类器添加到集合中
self.ensemble.append(classifier)
def predict(self, X):
# 使用投票机制进行预测
predictions = np.zeros((X.shape[0], self.n_estimators))
for i, classifier in enumerate(self.ensemble):
predictions[:, i] = classifier.predict(X)
return np.round(np.mean(predictions, axis=1))
```
使用示例:
```python
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# 创建一个示例数据集
X, y = make_classification(n_samples=1000, n_features=10, random_state=42)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 创建 EasyEnsemble 分类器并进行训练
ensemble = EasyEnsemble(n_estimators=10)
ensemble.fit(X_train, y_train)
# 进行预测并评估准确性
y_pred = ensemble.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
```
请注意,这只是一个简单的实现示例,EasyEnsemble 算法的完整实现可能涉及更多的参数选择、特征选择和模型优化技术。此外,你可以根据自己的需求进行修改和改进。
相关推荐
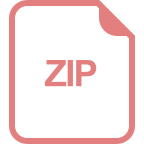
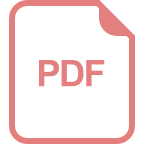
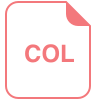
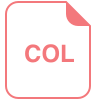
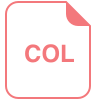
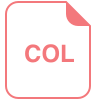
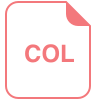
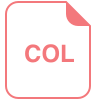
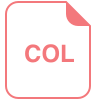
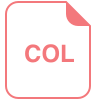
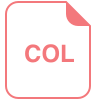
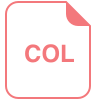
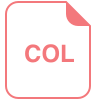