Android的opengl怎么导入
时间: 2023-12-05 16:31:25 浏览: 129
要在Android项目中使用OpenGL,需要在项目中添加OpenGL库文件和相关的JNI代码。下面是一些基本步骤:
1. 在 Android Studio 中打开你的项目,然后在项目结构中右键点击“app”文件夹并选择“New -> Folder -> JNI Folder”。
2. 选择“JNI Folder”的类型为“JNI Folder”,然后点击“Finish”。
3. 在“jni”文件夹下创建一个名为“native-lib.cpp”的文件,并将以下代码复制到该文件中:
```c++
#include <jni.h>
#include <string>
extern "C" JNIEXPORT jstring JNICALL
Java_com_example_myapplication_MainActivity_stringFromJNI(
JNIEnv* env,
jobject /* this */) {
std::string hello = "Hello from C++";
return env->NewStringUTF(hello.c_str());
}
```
4. 在“app”文件夹下打开“build.gradle”文件,并将以下代码添加到“dependencies”中:
```groovy
implementation 'com.android.support:appcompat-v7:28.0.0'
implementation 'com.android.support.constraint:constraint-layout:1.1.3'
implementation 'com.android.support:design:28.0.0'
implementation 'com.android.support:support-v4:28.0.0'
implementation 'com.android.support:multidex:1.0.3'
implementation 'com.google.android.gms:play-services-maps:16.0.0'
implementation 'com.google.android.gms:play-services-location:16.0.0'
implementation 'com.google.android.gms:play-services-ads:17.1.2'
implementation 'com.google.android.gms:play-services-auth:16.0.1'
implementation 'com.google.android.gms:play-services-drive:16.0.0'
implementation 'com.google.android.gms:play-services-games:16.0.0'
implementation 'com.google.android.gms:play-services-wearable:16.0.1'
implementation 'com.google.android.gms:play-services-plus:16.0.0'
implementation 'com.google.firebase:firebase-core:16.0.4'
implementation 'com.google.firebase:firebase-database:16.0.4'
implementation 'com.google.firebase:firebase-storage:16.0.4'
implementation 'com.google.firebase:firebase-auth:16.0.4'
implementation 'com.google.firebase:firebase-messaging:17.3.3'
implementation 'com.google.firebase:firebase-crash:16.0.3'
implementation 'com.google.firebase:firebase-config:16.0.0'
implementation 'com.google.firebase:firebase-perf:16.0.0'
implementation 'com.google.firebase:firebase-functions:16.1.0'
implementation 'com.google.firebase:firebase-appindexing:16.0.2'
implementation 'com.google.firebase:firebase-invites:16.0.4'
implementation 'com.google.firebase:firebase-ads:15.0.0'
implementation 'com.google.firebase:firebase-firestore:17.1.0'
implementation 'com.google.firebase:firebase-ml-vision:17.0.0'
implementation 'com.google.firebase:firebase-ml-vision-image-label-model:15.0.0'
implementation 'com.google.firebase:firebase-ml-vision-face-model:15.0.0'
implementation 'com.google.firebase:firebase-ml-model-interpreter:16.0.0'
implementation 'com.google.firebase:firebase-ml-natural-language:16.0.0'
implementation 'com.google.firebase:firebase-ml-vision-barcode-model:16.0.0'
implementation 'com.google.firebase:firebase-storage-ktx:16.0.4'
implementation 'com.google.firebase:firebase-database-ktx:16.0.4'
implementation 'org.jetbrains.kotlin:kotlin-stdlib-jdk7:1.3.11'
implementation 'com.github.bumptech.glide:glide:4.9.0'
kapt 'com.github.bumptech.glide:compiler:4.9.0'
implementation 'com.squareup.retrofit2:retrofit:2.5.0'
implementation 'com.squareup.retrofit2:converter-gson:2.5.0'
implementation 'com.squareup.okhttp3:logging-interceptor:3.12.1'
implementation 'com.squareup.okhttp3:okhttp:3.12.1'
implementation 'com.google.code.gson:gson:2.8.5'
implementation 'com.jakewharton.retrofit:retrofit2-kotlin-coroutines-adapter:0.9.2'
implementation 'com.facebook.android:facebook-login:4.38.1'
implementation 'com.facebook.android:facebook-share:4.38.1'
implementation 'io.branch.sdk.android:library:3.0.0'
implementation 'com.google.android:flexbox:1.0.0'
implementation 'com.google.code.findbugs:jsr305:3.0.2'
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:1.1.0'
implementation 'com.google.dagger:dagger:2.21'
kapt 'com.google.dagger:dagger-compiler:2.21'
implementation 'com.google.dagger:dagger-android:2.21'
implementation 'com.google.dagger:dagger-android-support:2.21'
kapt 'com.google.dagger:dagger-android-processor:2.21'
implementation 'com.google.android.material:material:1.0.0'
implementation 'com.squareup.picasso:picasso:2.71828'
```
5. 在“app”文件夹下打开“build.gradle”文件,并将以下代码添加到“defaultConfig”中:
```groovy
ndk {
abiFilters 'armeabi-v7a','arm64-v8a','x86','x86_64'
}
```
6. 将以下代码添加到“build.gradle”文件的末尾:
```groovy
externalNativeBuild {
cmake {
path "CMakeLists.txt"
}
}
```
7. 在“app”文件夹下创建一个名为“CMakeLists.txt”的文件,并将以下代码复制到该文件中:
```cmake
cmake_minimum_required(VERSION 3.4.1)
add_library( # Sets the name of the library.
native-lib
# Sets the library as a shared library.
SHARED
# Provides a relative path to your source file(s).
src/main/cpp/native-lib.cpp )
# Searches for a specified prebuilt library and stores the path as a
# variable. Because system libraries are included in the search path by
# default, you only need to specify the name of the public NDK library
# you want to add. CMake verifies that the library exists before
# completing its build.
find_library( # Sets the name of the path variable.
log-lib
# Specifies the name of the NDK library that
# you want CMake to locate.
log )
# Specifies libraries CMake should link to your target library. You
# can link multiple libraries, such as libraries you define in the
# same build script, prebuilt third-party libraries, or system libraries.
target_link_libraries( # Specifies the target library.
native-lib
# Links the target library to the log library
# included in the NDK.
${log-lib} )
```
8. 在“app”文件夹下创建一个名为“Android.mk”的文件,并将以下代码复制到该文件中:
```makefile
LOCAL_PATH := $(call my-dir)
include $(CLEAR_VARS)
# Here we give our module name and source file(s)
LOCAL_MODULE := native-lib
LOCAL_SRC_FILES := native-lib.cpp
include $(BUILD_SHARED_LIBRARY)
```
9. 在“app”文件夹下创建一个名为“Application.mk”的文件,并将以下代码复制到该文件中:
```makefile
APP_ABI := armeabi-v7a arm64-v8a x86 x86_64
APP_PLATFORM := android-19
```
10. 在“app”文件夹下创建一个名为“jniLibs”的文件夹,然后将你的OpenGL库文件复制到该文件夹中。你可以在 Android NDK 的“sources\platforms\android-xx\arch-xxx\usr\lib”目录下找到这些库文件。
完成上述步骤后,你就可以在 Android 应用程序中使用 OpenGL 了。
阅读全文
相关推荐
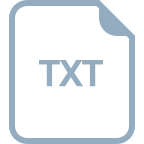

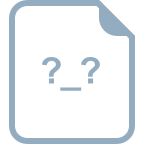
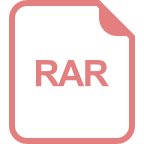
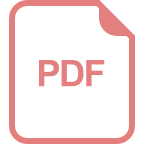
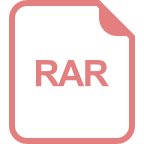
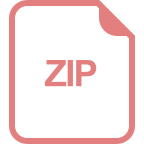
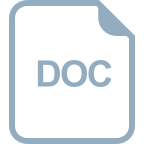
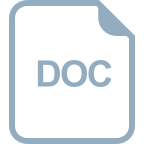
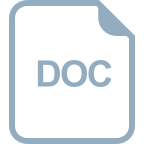
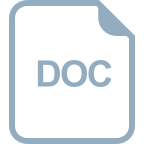
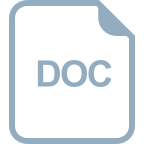
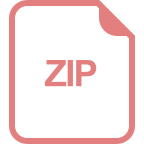
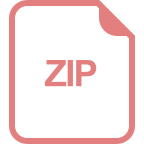
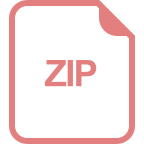