写一个将列表长度为3作为输入的branchynet代码
时间: 2023-12-14 22:39:09 浏览: 147
以下是一个示例的BranchyNet代码,它接受长度为3的列表作为输入,并在每个分支的末尾输出单个数字:
```python
import torch
import torch.nn as nn
from branchynet import BranchyNet
class MyBranchyNet(nn.Module):
def __init__(self):
super(MyBranchyNet, self).__init__()
# Define three branches
self.branch1 = nn.Sequential(
nn.Linear(3, 128),
nn.ReLU(),
nn.Linear(128, 1)
)
self.branch2 = nn.Sequential(
nn.Linear(3, 256),
nn.ReLU(),
nn.Linear(256, 1)
)
self.branch3 = nn.Sequential(
nn.Linear(3, 512),
nn.ReLU(),
nn.Linear(512, 1)
)
# Define the classifier
self.classifier = nn.Sequential(
nn.Linear(3, 64),
nn.ReLU(),
nn.Linear(64, 2),
nn.LogSoftmax(dim=1)
)
# Define the BranchyNet
self.branchyNet = BranchyNet(self.classifier, [self.branch1, self.branch2, self.branch3])
def forward(self, x):
# Pass the input through the BranchyNet
out = self.branchyNet(x)
# Return the output
return out
# Example usage
net = MyBranchyNet()
input = torch.tensor([1, 2, 3], dtype=torch.float32)
output = net(input.unsqueeze(0))
print(output)
```
在这个示例中,我们在`MyBranchyNet`中定义了三个分支,每个分支都包含两个全连接层和一个ReLU激活函数。然后,我们定义一个分类器,它将输入传递到三个分支中,并在每个分支的末尾输出单个数字。最后,我们使用`BranchyNet`类将分类器和分支列表组合在一起。
在`forward`方法中,我们将输入传递给`BranchyNet`,并返回输出。请注意,我们在输入张量上使用了`unsqueeze`方法,以将其从形状为`(3,)`的一维向量转换为形状为`(1, 3)`的二维张量,以便与神经网络模块兼容。
阅读全文
相关推荐












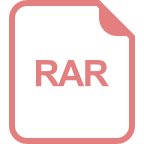

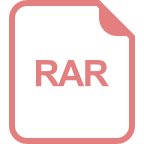