使用多线程和协程两种技术爬去豆瓣电影排行榜的电影名称和评分信息
时间: 2023-08-08 08:13:28 浏览: 179
使用多线程和协程两种技术可以提高爬取效率。以下是使用Python的多线程和协程爬取豆瓣电影排行榜的电影名称和评分信息的示例代码:
## 多线程
```python
import requests
from bs4 import BeautifulSoup
from concurrent.futures import ThreadPoolExecutor
# 定义线程池大小
THREAD_POOL_SIZE = 10
# 定义爬取函数
def spider(start):
url = 'https://movie.douban.com/top250?start=' + str(start)
# 发送请求
response = requests.get(url)
# 解析HTML代码
soup = BeautifulSoup(response.text, 'html.parser')
# 查找所有的电影条目
movie_items = soup.find_all('div', {'class': 'hd'})
# 遍历电影条目,获取电影名称和评分信息
for item in movie_items:
title = item.a.span.text.strip()
rating = item.parent.find('span', {'class': 'rating_num'}).text
print(title, rating)
# 使用线程池执行爬取任务
with ThreadPoolExecutor(THREAD_POOL_SIZE) as executor:
# 遍历每一页的电影列表
for i in range(0, 250, 25):
executor.submit(spider, i)
```
## 协程
```python
import asyncio
import aiohttp
from bs4 import BeautifulSoup
# 定义爬取函数
async def spider(start):
url = 'https://movie.douban.com/top250?start=' + str(start)
# 发送请求
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
html = await response.text()
# 解析HTML代码
soup = BeautifulSoup(html, 'html.parser')
# 查找所有的电影条目
movie_items = soup.find_all('div', {'class': 'hd'})
# 遍历电影条目,获取电影名称和评分信息
for item in movie_items:
title = item.a.span.text.strip()
rating = item.parent.find('span', {'class': 'rating_num'}).text
print(title, rating)
# 定义协程任务
async def main():
# 创建事件循环
loop = asyncio.get_event_loop()
# 创建协程任务列表
tasks = [loop.create_task(spider(i)) for i in range(0, 250, 25)]
# 执行协程任务
await asyncio.wait(tasks)
# 运行主函数
if __name__ == '__main__':
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
```
以上是多线程和协程两种技术爬取豆瓣电影排行榜的电影名称和评分信息的示例代码,供参考。
阅读全文
相关推荐
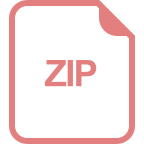
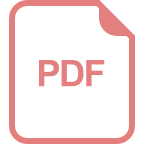
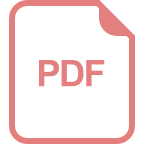















