For input string: "-4.00"
时间: 2023-12-03 16:04:27 浏览: 130
如果您正在使用Java编程语言,可以使用Double.parseDouble("-4.00")将字符串"-4.00"转换为double类型的数字-4.0。例如:
```java
String input = "-4.00";
double num = Double.parseDouble(input);
System.out.println(num); // 输出: -4.0
```
如果您使用其他编程语言,请查看该语言中字符串转换为数字的相关文档或函数。
相关问题
For input string: "4.00"
This input string represents a number with a decimal point, specifically the number four with two decimal places. In programming, this input string would typically need to be converted to a numerical data type, such as a float or a double, in order to perform mathematical operations with it. The specific method for doing so may vary depending on the programming language being used.
用C语言写基于栈的中缀算术表达式求值,例如输入2+2= 或20*(4.5-3)= 输出4.00 30.00
你好!感谢你的提问。关于基于栈的中缀算术表达式求值,可以使用以下步骤:
1. 创建两个栈:一个用于存储操作数、一个用于存储操作符;
2. 从左到右扫描中缀表达式,遇到数字直接入操作数栈;
3. 遇到操作符时,如果操作符栈为空或者栈顶操作符是左括号,则直接入操作符栈;否则,将栈顶操作符弹出,并将操作数栈中的两个操作数弹出,进行计算,并将结果入操作数栈,直到操作符栈为空或者栈顶操作符是左括号,然后将当前操作符入操作符栈;
4. 遇到左括号直接入操作符栈;
5. 遇到右括号时,将操作符栈中的操作符弹出,直到遇到左括号,将其丢弃;
6. 扫描完成后,如果操作符栈中还有操作符,则取出操作数栈中的两个操作数进行计算,直到操作符栈为空。
下面是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <string.h>
#define MAXSIZE 1024
typedef struct {
double data[MAXSIZE];
int top;
} OpndStack;
typedef struct {
char data[MAXSIZE];
int top;
} OprtStack;
void InitStack(OpndStack *s) {
s->top = -1;
}
void Push(OpndStack *s, double x) {
if (s->top == MAXSIZE - 1) {
printf("Stack overflow!\n");
exit(1);
} else {
s->top++;
s->data[s->top] = x;
}
}
double Pop(OpndStack *s) {
if (s->top == -1) {
printf("Stack underflow!\n");
exit(1);
} else {
double x = s->data[s->top];
s->top--;
return x;
}
}
double GetTop(OpndStack *s) {
if (s->top == -1) {
printf("Stack is empty!\n");
exit(1);
} else {
return s->data[s->top];
}
}
void InitStack2(OprtStack *s) {
s->top = -1;
}
void Push2(OprtStack *s, char x) {
if (s->top == MAXSIZE - 1) {
printf("Stack overflow!\n");
exit(1);
} else {
s->top++;
s->data[s->top] = x;
}
}
char Pop2(OprtStack *s) {
if (s->top == -1) {
printf("Stack underflow!\n");
exit(1);
} else {
char x = s->data[s->top];
s->top--;
return x;
}
}
char GetTop2(OprtStack *s) {
if (s->top == -1) {
printf("Stack is empty!\n");
exit(1);
} else {
return s->data[s->top];
}
}
int IsEmpty(OpndStack *s) {
return s->top == -1 ? 1 : 0;
}
int IsEmpty2(OprtStack *s) {
return s->top == -1 ? 1 : 0;
}
int Priority(char oprt) {
switch (oprt) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '(':
case ')':
return 0;
default:
return -1;
}
}
void Calculate(OpndStack *s, char oprt) {
double opnd1, opnd2, result;
opnd2 = Pop(s);
opnd1 = Pop(s);
switch (oprt) {
case '+':
result = opnd1 + opnd2;
break;
case '-':
result = opnd1 - opnd2;
break;
case '*':
result = opnd1 * opnd2;
break;
case '/':
result = opnd1 / opnd2;
break;
default:
printf("Invalid operator!\n");
exit(1);
}
Push(s, result);
}
double EvaluateExpression(char *s) {
OpndStack opndStack;
OprtStack oprtStack;
double num = 0.0, result;
int i = 0, flag = 0;
InitStack(&opndStack);
InitStack2(&oprtStack);
while (s[i]) {
if (isdigit(s[i])) {
flag = 1;
num = num * 10 + s[i] - '0';
i++;
} else {
if (flag) {
Push(&opndStack, num);
num = 0.0;
flag = 0;
}
if (s[i] == ')') {
while (GetTop2(&oprtStack) != '(') {
Calculate(&opndStack, Pop2(&oprtStack));
}
Pop2(&oprtStack);
} else {
while (!IsEmpty2(&oprtStack) && Priority(s[i]) <= Priority(GetTop2(&oprtStack))) {
Calculate(&opndStack, Pop2(&oprtStack));
}
Push2(&oprtStack, s[i]);
i++;
}
}
}
if (flag) {
Push(&opndStack, num);
}
while (!IsEmpty2(&oprtStack)) {
Calculate(&opndStack, Pop2(&oprtStack));
}
result = Pop(&opndStack);
return result;
}
int main() {
char s[MAXSIZE];
double result;
printf("Please input an infix arithmetic expression:\n");
fgets(s, MAXSIZE, stdin);
s[strlen(s) - 1] = '\0';
result = EvaluateExpression(s);
printf("%.2f", result);
return 0;
}
```
你可以输入2 2= 或20*(4.5-3)= 测试一下。
阅读全文
相关推荐
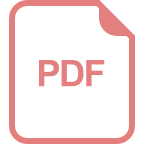
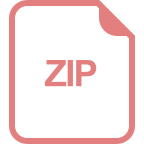
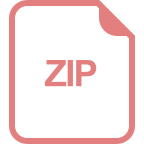
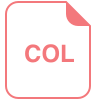
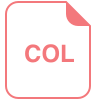
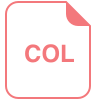
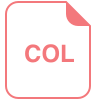
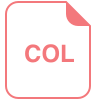
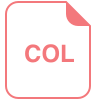
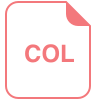
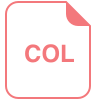
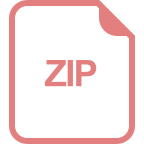