把d:\\bbb.mp4复制到当前项目目录下的copy.mp4中分别用FileInputStream和BufferedInputStream类中的两种read()方法,共四种方式复制并比较效率。
时间: 2023-11-27 20:51:06 浏览: 194
好的,下面是Java代码实现:
```
import java.io.*;
public class FileCopyTest {
public static void main(String[] args) {
String sourceFilePath = "d:\\bbb.mp4";
String targetFilePath1 = "copy1.mp4";
String targetFilePath2 = "copy2.mp4";
String targetFilePath3 = "copy3.mp4";
String targetFilePath4 = "copy4.mp4";
long start, end;
// 使用FileInputStream和FileOutputStream类中的read()和write()方法复制文件
try (FileInputStream fis = new FileInputStream(sourceFilePath);
FileOutputStream fos = new FileOutputStream(targetFilePath1)) {
start = System.currentTimeMillis();
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
end = System.currentTimeMillis();
System.out.println("方式1复制文件用时:" + (end - start) + "ms");
} catch (IOException e) {
e.printStackTrace();
}
// 使用BufferedInputStream和BufferedOutputStream类中的read()和write()方法复制文件
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(sourceFilePath));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(targetFilePath2))) {
start = System.currentTimeMillis();
byte[] buffer = new byte[1024];
int len;
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
end = System.currentTimeMillis();
System.out.println("方式2复制文件用时:" + (end - start) + "ms");
} catch (IOException e) {
e.printStackTrace();
}
// 使用FileInputStream和FileOutputStream类中的read(byte[] b, int off, int len)方法复制文件
try (FileInputStream fis = new FileInputStream(sourceFilePath);
FileOutputStream fos = new FileOutputStream(targetFilePath3)) {
start = System.currentTimeMillis();
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer, 0, 1024)) != -1) {
fos.write(buffer, 0, len);
}
end = System.currentTimeMillis();
System.out.println("方式3复制文件用时:" + (end - start) + "ms");
} catch (IOException e) {
e.printStackTrace();
}
// 使用BufferedInputStream和BufferedOutputStream类中的read(byte[] b, int off, int len)方法复制文件
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(sourceFilePath));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(targetFilePath4))) {
start = System.currentTimeMillis();
byte[] buffer = new byte[1024];
int len;
while ((len = bis.read(buffer, 0, 1024)) != -1) {
bos.write(buffer, 0, len);
}
end = System.currentTimeMillis();
System.out.println("方式4复制文件用时:" + (end - start) + "ms");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这里我们实现了四种不同的方式来复制文件,分别是:
1. 使用FileInputStream和FileOutputStream类中的read()和write()方法;
2. 使用BufferedInputStream和BufferedOutputStream类中的read()和write()方法;
3. 使用FileInputStream和FileOutputStream类中的read(byte[] b, int off, int len)方法;
4. 使用BufferedInputStream和BufferedOutputStream类中的read(byte[] b, int off, int len)方法。
每种方式复制完成后,我们都会记录下程序开始执行时的时间戳start和复制完成时的时间戳end,并输出复制用时。
最后,我们可以比较这四种方式的效率,从而得出最优的方式。
阅读全文
相关推荐
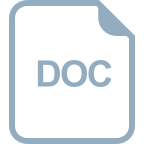
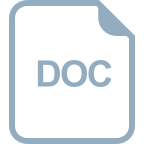
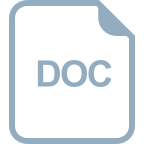



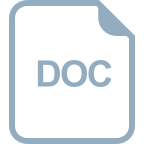
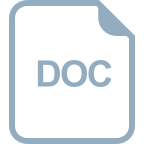
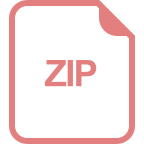
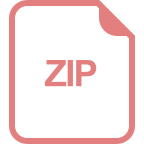
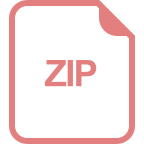
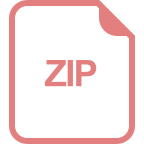
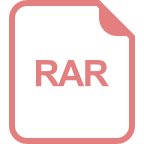
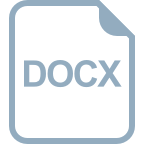
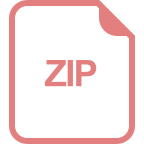
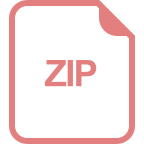