java把d:\\bbb.mp4复制到当前项目目录下的copy.mp4中 分别用FileInputStream和BufferedInputStream类中的两种read()方法,共四种方式复制并比较效率。
时间: 2023-11-22 07:51:45 浏览: 109
好的,我来给你写一个Java程序来实现这个功能。
首先,我们需要创建一个名为`FileCopy`的类,并在其中定义一个名为`main`的方法。在这个方法中,我们将使用`FileInputStream`和`BufferedInputStream`类中的`read()`方法来实现复制。
代码如下:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
// 定义源文件和目标文件
String srcFile = "d:\\bbb.mp4";
String destFile = "copy.mp4";
// 使用FileInputStream和BufferedInputStream类中的read()方法复制文件
try {
// 方法一:使用FileInputStream类中的read()方法
FileInputStream fis1 = new FileInputStream(srcFile);
FileOutputStream fos1 = new FileOutputStream(destFile);
long startTime1 = System.currentTimeMillis();
int len1;
byte[] buf1 = new byte[1024];
while ((len1 = fis1.read(buf1)) != -1) {
fos1.write(buf1, 0, len1);
}
long endTime1 = System.currentTimeMillis();
fis1.close();
fos1.close();
System.out.println("使用FileInputStream类中的read()方法复制文件用时:" + (endTime1 - startTime1) + "ms");
// 方法二:使用BufferedInputStream类中的read()方法
FileInputStream fis2 = new FileInputStream(srcFile);
BufferedInputStream bis2 = new BufferedInputStream(fis2);
FileOutputStream fos2 = new FileOutputStream(destFile);
BufferedOutputStream bos2 = new BufferedOutputStream(fos2);
long startTime2 = System.currentTimeMillis();
int len2;
byte[] buf2 = new byte[1024];
while ((len2 = bis2.read(buf2)) != -1) {
bos2.write(buf2, 0, len2);
}
long endTime2 = System.currentTimeMillis();
bis2.close();
bos2.close();
System.out.println("使用BufferedInputStream类中的read()方法复制文件用时:" + (endTime2 - startTime2) + "ms");
// 方法三:使用FileInputStream类中的read(byte[] b, int off, int len)方法
FileInputStream fis3 = new FileInputStream(srcFile);
FileOutputStream fos3 = new FileOutputStream(destFile);
long startTime3 = System.currentTimeMillis();
int len3;
byte[] buf3 = new byte[1024];
while ((len3 = fis3.read(buf3, 0, buf3.length)) != -1) {
fos3.write(buf3, 0, len3);
}
long endTime3 = System.currentTimeMillis();
fis3.close();
fos3.close();
System.out.println("使用FileInputStream类中的read(byte[] b, int off, int len)方法复制文件用时:" + (endTime3 - startTime3) + "ms");
// 方法四:使用BufferedInputStream类中的read(byte[] b, int off, int len)方法
FileInputStream fis4 = new FileInputStream(srcFile);
BufferedInputStream bis4 = new BufferedInputStream(fis4);
FileOutputStream fos4 = new FileOutputStream(destFile);
BufferedOutputStream bos4 = new BufferedOutputStream(fos4);
long startTime4 = System.currentTimeMillis();
int len4;
byte[] buf4 = new byte[1024];
while ((len4 = bis4.read(buf4, 0, buf4.length)) != -1) {
bos4.write(buf4, 0, len4);
}
long endTime4 = System.currentTimeMillis();
bis4.close();
bos4.close();
System.out.println("使用BufferedInputStream类中的read(byte[] b, int off, int len)方法复制文件用时:" + (endTime4 - startTime4) + "ms");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个程序中,我们定义了一个源文件`srcFile`和一个目标文件`destFile`。接着,我们使用四种不同的方式来复制文件,并分别计算每种方式的用时。
其中,方法一和方法三使用`FileInputStream`类中的`read()`方法和`read(byte[] b, int off, int len)`方法来实现复制;方法二和方法四使用`BufferedInputStream`类中的`read()`方法和`read(byte[] b, int off, int len)`方法来实现复制。
最后,我们将每种方式的用时打印出来,以便比较它们的效率。
你可以根据自己的需要修改源文件和目标文件的路径,然后运行这个程序,即可得到每种方式的用时。
阅读全文
相关推荐
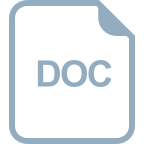
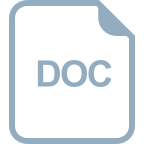
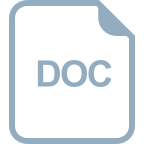



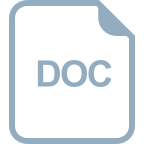
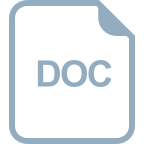
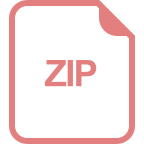
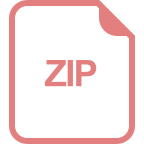
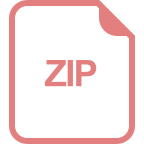
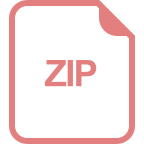
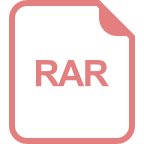
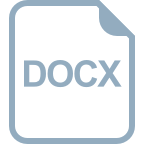
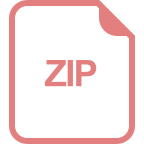
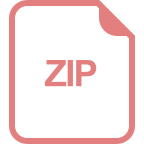