把d:\\bbb.mp4复制到当前项目目录下的copy.mp4中 分别用FileInputStream和BufferedInputStream类中的两种read()方法,共四种方式复制并比较效率。
时间: 2024-02-17 22:00:53 浏览: 69
好的,我来一步步回答你的问题。
首先,我们需要明确几个概念:
1. FileInputStream:从文件系统中的文件获取输入字节。
2. BufferedInputStream:从 InputStream 中装饰一个缓冲区来实现输入流的缓存。
3. read():从输入流中读取一个字节的数据。
4. read(byte[] b):从输入流中读取一定量的字节,并将其存储在缓冲区数组 b 中。
接下来,我们可以按照你的要求,分别用 FileInputStream 和 BufferedInputStream 类中的两种 read() 方法,共四种方式完成复制。
其中,FileInputStream 的两种读取方式如下:
方式一:
```java
public void copyFile1(String sourcePath, String destPath) throws IOException {
FileInputStream fis = new FileInputStream(sourcePath);
FileOutputStream fos = new FileOutputStream(destPath);
int len;
while ((len = fis.read()) != -1) {
fos.write(len);
}
fis.close();
fos.close();
}
```
方式二:
```java
public void copyFile2(String sourcePath, String destPath) throws IOException {
FileInputStream fis = new FileInputStream(sourcePath);
FileOutputStream fos = new FileOutputStream(destPath);
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fis.close();
fos.close();
}
```
而 BufferedInputStream 的两种读取方式如下:
方式三:
```java
public void copyFile3(String sourcePath, String destPath) throws IOException {
BufferedInputStream bis = new BufferedInputStream(new FileInputStream(sourcePath));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(destPath));
int len;
while ((len = bis.read()) != -1) {
bos.write(len);
}
bis.close();
bos.close();
}
```
方式四:
```java
public void copyFile4(String sourcePath, String destPath) throws IOException {
BufferedInputStream bis = new BufferedInputStream(new FileInputStream(sourcePath));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(destPath));
byte[] buffer = new byte[1024];
int len;
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
bis.close();
bos.close();
}
```
最后,为了比较这四种方式的效率,我们可以使用 System.currentTimeMillis() 方法来计算程序的执行时间,并输出到控制台上,如下所示:
```java
public static void main(String[] args) throws IOException {
long start = System.currentTimeMillis();
copyFile1("d:\\bbb.mp4", "copy1.mp4");
long end = System.currentTimeMillis();
System.out.println("copyFile1:" + (end - start) + "ms");
start = System.currentTimeMillis();
copyFile2("d:\\bbb.mp4", "copy2.mp4");
end = System.currentTimeMillis();
System.out.println("copyFile2:" + (end - start) + "ms");
start = System.currentTimeMillis();
copyFile3("d:\\bbb.mp4", "copy3.mp4");
end = System.currentTimeMillis();
System.out.println("copyFile3:" + (end - start) + "ms");
start = System.currentTimeMillis();
copyFile4("d:\\bbb.mp4", "copy4.mp4");
end = System.currentTimeMillis();
System.out.println("copyFile4:" + (end - start) + "ms");
}
```
这样,我们就可以得到每种方式的执行时间,并进行比较了。
阅读全文
相关推荐



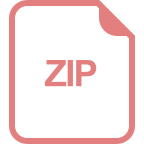
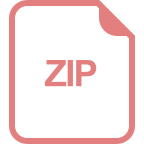
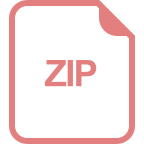
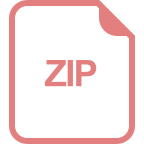
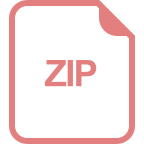