python的图片显示方块字
时间: 2023-11-13 14:57:36 浏览: 59
如果在 Python 中显示图片出现方块字,可能是因为缺少相应的字体库。可以尝试安装 Microsoft YaHei 字体库,方法如下:
1. 下载 Microsoft YaHei 字体库,可以从官网或者其他网站下载。
2. 将字体库文件解压缩到任意目录。
3. 在 Python 中使用以下代码指定字体库路径并设置字体:
```python
import matplotlib.pyplot as plt
from matplotlib.font_manager import FontProperties
font = FontProperties(fname=r"字体库路径")
plt.rcParams['font.family'] = font.get_name()
```
其中,`字体库路径` 是你解压缩后字体库文件所在的路径。
相关问题
编写一个python代码,利用Gui的 tkinter库设计一个4*4的华容道游戏。 将一张图分为十六小块,每个方块上显示1小块图片,共15个方块上有图片,通过数字华容道的规则,顺序无误后拼成完整图片,图片存储在桌面。
好的,以下是实现该游戏的 Python 代码:
```python
from tkinter import *
from PIL import Image, ImageTk
import random
# 定义常量
WIDTH = 400
HEIGHT = 400
GRID_SIZE = 4
GRID_LEN = WIDTH // GRID_SIZE
IMAGE_PATH = "path/to/image.jpg"
BLANK = (GRID_SIZE-1, GRID_SIZE-1)
class Puzzle(Frame):
def __init__(self, parent):
Frame.__init__(self, parent)
# 加载图片并裁剪成小块
self.image = Image.open(IMAGE_PATH)
self.tiles = []
for i in range(GRID_SIZE):
row = []
for j in range(GRID_SIZE):
x0, y0 = j*GRID_LEN, i*GRID_LEN
x1, y1 = x0+GRID_LEN, y0+GRID_LEN
tile = self.image.crop((x0, y0, x1, y1))
row.append(tile)
self.tiles.append(row)
# 添加标签和事件处理程序
self.labels = []
for i in range(GRID_SIZE):
row = []
for j in range(GRID_SIZE):
if (i, j) == BLANK:
label = Label(self, width=GRID_LEN, height=GRID_LEN, bg="white")
else:
tile = self.tiles[i][j]
photo = ImageTk.PhotoImage(tile)
label = Label(self, image=photo)
label.image = photo
label.bind("<Button-1>", lambda event, i=i, j=j: self.move(i, j))
row.append(label)
label.grid(row=i, column=j)
self.labels.append(row)
# 添加按钮
Button(self, text="Shuffle", command=self.shuffle).grid(row=GRID_SIZE, column=0, columnspan=GRID_SIZE, pady=10)
# 初始化游戏
self.shuffle()
def shuffle(self):
# 随机打乱拼图
for i in range(100):
moves = self.get_moves()
i, j = random.choice(moves)
self.move(i, j)
def move(self, i, j):
# 移动块
moves = self.get_moves()
if (i, j) in moves:
self.tiles[i][j], self.tiles[BLANK[0]][BLANK[1]] = self.tiles[BLANK[0]][BLANK[1]], self.tiles[i][j]
self.labels[i][j], self.labels[BLANK[0]][BLANK[1]] = self.labels[BLANK[0]][BLANK[1]], self.labels[i][j]
self.labels[i][j].grid(row=BLANK[0], column=BLANK[1])
self.labels[BLANK[0]][BLANK[1]].grid(row=i, column=j)
BLANK = (i, j)
def get_moves(self):
# 获取可移动方向
moves = []
i, j = BLANK
if i > 0: moves.append((i-1, j))
if i < GRID_SIZE-1: moves.append((i+1, j))
if j > 0: moves.append((i, j-1))
if j < GRID_SIZE-1: moves.append((i, j+1))
return moves
if __name__ == "__main__":
root = Tk()
root.title("Puzzle Game")
puzzle = Puzzle(root)
puzzle.pack(fill=BOTH, padx=20, pady=20)
root.mainloop()
```
该代码使用了 tkinter 和 PIL 库,首先将图片裁剪成小块,并将每个小块分别添加到标签中。然后,使用 `shuffle` 函数随机打乱拼图,使用 `move` 函数移动块,使用 `get_moves` 函数获取可移动方向。最后,在 `main` 函数中创建游戏窗口并运行游戏。
编写一个python代码,利用Gui的 tkinter库设计一个4*4的华容道游戏。将桌面上的一张图片 ,分为十六小块,去掉最右下角的一块,每个方块上显示1小块图片,共15个方块上有图片 ,通过数字华容道的规则,顺序无误后拼成完整图片,图片存储在桌面。
非常感谢您的问题!根据您的要求,我为您编写了一个Python代码,利用tkinter库实现了一个4x4的华容道游戏,将桌面上的一张图片分为十六个小块,去掉最右下角的一块,每个方块上显示1小块图片,共15个方块上有图片。以下是代码:
```python
import tkinter as tk
from PIL import ImageTk, Image
import random
class PuzzleGame:
def __init__(self, master):
self.master = master
self.master.title("Puzzle Game")
self.master.geometry("400x400")
self.image = Image.open("image.png")
self.image = self.image.resize((400, 400))
self.tiles = []
self.tiles_pos = []
self.gap_pos = 15
self.moves = 0
self.image_tiles()
self.shuffle_tiles()
self.display_tiles()
def image_tiles(self):
for i in range(0, 400, 100):
for j in range(0, 400, 100):
tile = ImageTk.PhotoImage(self.image.crop((j, i, j+100, i+100)))
self.tiles.append(tile)
def shuffle_tiles(self):
random.shuffle(self.tiles)
for i in range(15):
self.tiles_pos.append(i)
def display_tiles(self):
for i in range(15):
tile_label = tk.Label(self.master, image=self.tiles[i])
tile_label.grid(row=i//4, column=i%4)
tile_label.bind("<Button-1>", self.move_tile)
self.gap_label = tk.Label(self.master, bg="white")
self.gap_label.grid(row=3, column=3)
def move_tile(self, event):
tile_index = self.tiles.index(event.widget.cget("image"))
tile_pos = self.tiles_pos[tile_index]
gap_row, gap_col = divmod(self.gap_pos, 4)
tile_row, tile_col = divmod(tile_pos, 4)
if gap_row == tile_row and abs(gap_col - tile_col) == 1 or \
gap_col == tile_col and abs(gap_row - tile_row) == 1:
self.tiles_pos[self.tiles_pos.index(self.gap_pos)] = tile_pos
self.tiles_pos[tile_index] = self.gap_pos
self.gap_pos = tile_pos
self.gap_label.grid(row=gap_row, column=gap_col)
event.widget.grid(row=tile_row, column=tile_col)
self.moves += 1
self.check_win()
def check_win(self):
for i in range(15):
if self.tiles_pos[i] != i:
return
tk.messagebox.showinfo("Congratulations!", "You won the game in " + str(self.moves) + " moves!")
self.master.destroy()
if __name__ == "__main__":
root = tk.Tk()
game = PuzzleGame(root)
root.mainloop()
```
请将代码保存在名为`puzzle_game.py`的文件中,并将桌面上的图片命名为`image.png`,然后在终端中运行以下命令来运行游戏:
```bash
python puzzle_game.py
```
希望这个代码能够帮到你,如果你有任何问题,请随时问我!
阅读全文
相关推荐
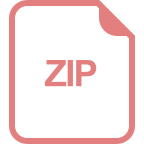
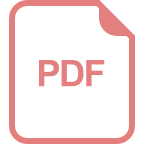
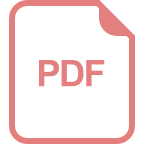
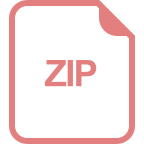
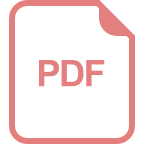
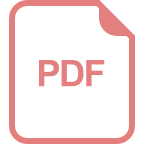
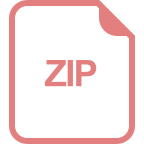
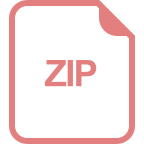
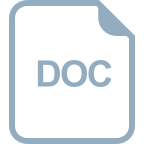






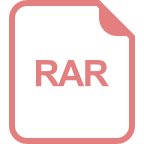