idea写个简单的jsp项目
时间: 2023-10-07 15:04:20 浏览: 47
好的,我可以为您提供一个简单的JSP项目的示例,该项目是一个简单的用户登录系统。以下是项目的详细说明:
1. 创建一个名为 "LoginSystem" 的 Web 项目。
2. 在 "src" 文件夹下创建一个名为 "com.loginsystem" 的包。
3. 在该包下创建一个名为 "LoginServlet" 的 Servlet。该Servlet用于处理用户登录请求,并验证用户输入的用户名和密码是否正确。
4. 在 "WebContent" 文件夹下创建一个名为 "login.jsp" 的 JSP 页面。该页面包含一个表单,用于接收用户输入的用户名和密码,并将其提交到 "LoginServlet" 中进行处理。
5. 在 "WebContent" 文件夹下创建一个名为 "home.jsp" 的 JSP 页面。该页面是用户登录成功后跳转的页面,包含一个简单的欢迎消息,以及用户信息的显示。
下面是示例代码:
LoginServlet.java
```
package com.loginsystem;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/LoginServlet")
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
// 在这里进行用户名和密码验证
// 如果用户名和密码正确,则将用户信息存储在 Session 中,并跳转到 home.jsp 页面
// 如果用户名和密码不正确,则返回 login.jsp 页面,并显示错误消息
if (username.equals("admin") && password.equals("admin123")) {
request.getSession().setAttribute("username", username);
response.sendRedirect("home.jsp");
} else {
request.setAttribute("error", "用户名或密码错误!");
request.getRequestDispatcher("login.jsp").forward(request, response);
}
}
}
```
login.jsp
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>用户登录</title>
</head>
<body>
<h1>用户登录</h1>
<%-- 显示错误消息 --%>
<p style="color: red">${error}</p>
<%-- 用户登录表单 --%>
<form method="post" action="LoginServlet">
<label>用户名:</label>
<input type="text" name="username"><br>
<label>密码:</label>
<input type="password" name="password"><br>
<input type="submit" value="登录">
</form>
</body>
</html>
```
home.jsp
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>欢迎</title>
</head>
<body>
<%-- 获取 Session 中的用户信息 --%>
<h1>欢迎,${sessionScope.username}!</h1>
<p>您已成功登录!</p>
</body>
</html>
```
希望这个示例能帮助到您。
相关推荐
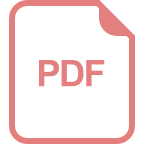
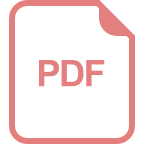














